mirror of
https://github.com/iv-org/videojs-quality-selector.git
synced 2025-04-25 17:59:16 -04:00
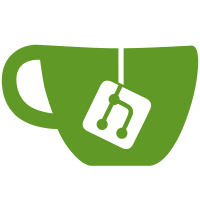
As things turn out, the middleware constructor is called every time `setSource`
is called [1]. This was causing a new listener to get bound to
`QUALITY_SELECTED` on each change by the quality selector. :( This change makes
it so the listener is only bound on the initial creation of the player.
[1] 03529163b6/src/js/tech/middleware.js (L66)
46 lines
1.5 KiB
JavaScript
46 lines
1.5 KiB
JavaScript
'use strict';
|
|
|
|
var _ = require('underscore');
|
|
|
|
module.exports = function(videojs) {
|
|
|
|
videojs.use('*', function(player) {
|
|
|
|
return {
|
|
|
|
setSource: function(playerSelectedSource, next) {
|
|
var sources = player.currentSources(),
|
|
userSelectedSource, chosenSource,
|
|
qualitySelector;
|
|
|
|
// There are generally two source options, the one that videojs
|
|
// auto-selects and the one that a "user" of this plugin has
|
|
// supplied via the `selected` property. `selected` can come from
|
|
// either the `<source>` tag or the list of sources passed to
|
|
// videojs using `src()`.
|
|
|
|
userSelectedSource = _.find(sources, function(source) {
|
|
// Must check for both boolean and string 'true' as sources set
|
|
// programmatically should use a boolean, but those coming from
|
|
// a `<source>` tag will use a string.
|
|
return source.selected === true || source.selected === 'true';
|
|
});
|
|
|
|
chosenSource = userSelectedSource || playerSelectedSource;
|
|
|
|
// Update the quality selector with the new source
|
|
qualitySelector = player.controlBar.getChild('qualitySelector');
|
|
if (qualitySelector) {
|
|
qualitySelector.setSelectedSource(chosenSource);
|
|
}
|
|
|
|
// Pass along the chosen source
|
|
next(null, chosenSource);
|
|
},
|
|
|
|
};
|
|
|
|
});
|
|
|
|
};
|