mirror of
https://github.com/SchildiChat/element-web.git
synced 2024-10-01 01:26:12 -04:00
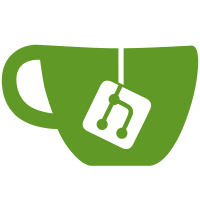
This uses the github.com:googlei18n/region-flags and imagemagick to generate 27x27 flag pngs. The flags have a 1px #e0e0e0 border and transparent padding such that each flag is of the same height (17px including the border).
85 lines
2.2 KiB
JavaScript
Executable File
85 lines
2.2 KiB
JavaScript
Executable File
#!/usr/bin/env node
|
|
|
|
// copies the resources into the webapp directory.
|
|
//
|
|
|
|
// cpx includes globbed parts of the filename in the destination, but excludes
|
|
// common parents. Hence, "res/{a,b}/**": the output will be "dest/a/..." and
|
|
// "dest/b/...".
|
|
const COPY_LIST = [
|
|
["res/manifest.json", "webapp"],
|
|
["res/{media,vector-icons}/**", "webapp"],
|
|
["res/flags/*", "webapp/flags/"],
|
|
["src/skins/vector/{fonts,img}/**", "webapp"],
|
|
["node_modules/emojione/assets/svg/*", "webapp/emojione/svg/"],
|
|
["node_modules/emojione/assets/png/*", "webapp/emojione/png/"],
|
|
["./config.json", "webapp", {directwatch: 1}],
|
|
];
|
|
|
|
const parseArgs = require('minimist');
|
|
const Cpx = require('cpx');
|
|
const chokidar = require('chokidar');
|
|
|
|
const argv = parseArgs(
|
|
process.argv.slice(2), {}
|
|
);
|
|
|
|
var watch = argv.w;
|
|
var verbose = argv.v;
|
|
|
|
function errCheck(err) {
|
|
if (err) {
|
|
console.error(err.message);
|
|
process.exit(1);
|
|
}
|
|
}
|
|
|
|
function next(i, err) {
|
|
errCheck(err);
|
|
|
|
if (i >= COPY_LIST.length) {
|
|
return;
|
|
}
|
|
|
|
const ent = COPY_LIST[i];
|
|
const source = ent[0];
|
|
const dest = ent[1];
|
|
const opts = ent[2] || {};
|
|
|
|
const cpx = new Cpx.Cpx(source, dest);
|
|
|
|
if (verbose) {
|
|
cpx.on("copy", (event) => {
|
|
console.log(`Copied: ${event.srcPath} --> ${event.dstPath}`);
|
|
});
|
|
cpx.on("remove", (event) => {
|
|
console.log(`Removed: ${event.path}`);
|
|
});
|
|
}
|
|
|
|
const cb = (err) => {next(i+1, err)};
|
|
|
|
if (watch) {
|
|
if (opts.directwatch) {
|
|
// cpx -w creates a watcher for the parent of any files specified,
|
|
// which in the case of config.json is '.', which inevitably takes
|
|
// ages to crawl. So we create our own watcher on the files
|
|
// instead.
|
|
const copy = () => {cpx.copy(errCheck)};
|
|
chokidar.watch(source)
|
|
.on('add', copy)
|
|
.on('change', copy)
|
|
.on('ready', cb)
|
|
.on('error', errCheck);
|
|
} else {
|
|
cpx.on('watch-ready', cb);
|
|
cpx.on("watch-error", cb);
|
|
cpx.watch();
|
|
}
|
|
} else {
|
|
cpx.copy(cb);
|
|
}
|
|
}
|
|
|
|
next(0);
|