mirror of
https://github.com/matrix-org/mjolnir.git
synced 2024-06-28 23:52:06 +00:00
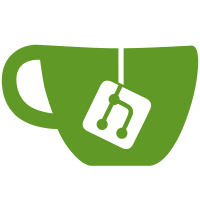
* Attempt to factor out protected rooms from Mjolnir. This is useful to the appservice because it means we don't have to wrap a Mjolnir that is designed to sync. It's also useful if we later on want to have specific settings per space. It's also just a nice seperation between Mjolnir's needs while syncing via client-server and the behaviour of syncing policy rooms. ### Things that have changed - `ErrorCache` no longer a static class (phew), gets used by `ProtectedRooms`. - `ManagementRoomOutput` class gets created to handle logging back to the management room. - Responsibilities for syncing member bans and server ACL are handled by `ProtectedRooms`. - Responsibilities for watched lists should be moved to `ProtectedRooms` if they haven't been. - `EventRedactionQueue` is moved to `ProtectedRooms` since this needs to happen after member bans. - ApplyServerAcls moved to `ProtectedRooms` - ApplyMemberBans move to `ProtectedRooms` - `logMessage` and `replaceRoomIdsWithPills` moved to `ManagementRoomOutput`. - `resyncJoinedRooms` has been made a little more clear, though I am concerned about how often it does run because it does seem expensive. * ProtectedRooms is not supposed to track joined rooms. The reason is because it is supposed to represent a specific set of rooms to protect, not do horrible logic for working out what rooms mjolnir is supposed to protect.
48 lines
2.5 KiB
TypeScript
48 lines
2.5 KiB
TypeScript
import { Mjolnir } from "../../src/Mjolnir";
|
|
import { IProtection } from "../../src/protections/IProtection";
|
|
import { newTestUser } from "./clientHelper";
|
|
|
|
describe("Test: Report polling", function() {
|
|
let client;
|
|
this.beforeEach(async function () {
|
|
client = await newTestUser(this.config.homeserverUrl, { name: { contains: "protection-settings" }});
|
|
})
|
|
it("Mjolnir correctly retrieves a report from synapse", async function() {
|
|
this.timeout(40000);
|
|
|
|
let protectedRoomId = await this.mjolnir.client.createRoom({ invite: [await client.getUserId()] });
|
|
await client.joinRoom(protectedRoomId);
|
|
await this.mjolnir.addProtectedRoom(protectedRoomId);
|
|
|
|
const eventId = await client.sendMessage(protectedRoomId, {msgtype: "m.text", body: "uwNd3q"});
|
|
await new Promise(async resolve => {
|
|
await this.mjolnir.protectionManager.registerProtection(new class implements IProtection {
|
|
name = "jYvufI";
|
|
description = "A test protection";
|
|
settings = { };
|
|
handleEvent = async (mjolnir: Mjolnir, roomId: string, event: any) => { };
|
|
handleReport = (mjolnir: Mjolnir, roomId: string, reporterId: string, event: any, reason?: string) => {
|
|
if (reason === "x5h1Je") {
|
|
resolve(null);
|
|
}
|
|
};
|
|
});
|
|
await this.mjolnir.protectionManager.enableProtection("jYvufI");
|
|
await client.doRequest(
|
|
"POST",
|
|
`/_matrix/client/r0/rooms/${encodeURIComponent(protectedRoomId)}/report/${encodeURIComponent(eventId)}`, "", {
|
|
reason: "x5h1Je"
|
|
}
|
|
);
|
|
});
|
|
// So I kid you not, it seems like we can quit before the webserver for reports sends a respond to the client (via L#26)
|
|
// because the promise above gets resolved before we finish awaiting the report sending request on L#31,
|
|
// then mocha's cleanup code runs (and shuts down the webserver) before the webserver can respond.
|
|
// Wait a minute 😲😲🤯 it's not even supposed to be using the webserver if this is testing report polling.
|
|
// Ok, well apparently that needs a big refactor to change, but if you change the config before running this test,
|
|
// then you can ensure that report polling works. https://github.com/matrix-org/mjolnir/issues/326.
|
|
await new Promise(resolve => setTimeout(resolve, 1000));
|
|
});
|
|
});
|
|
|