mirror of
https://mau.dev/maunium/synapse.git
synced 2024-07-16 08:01:58 +00:00
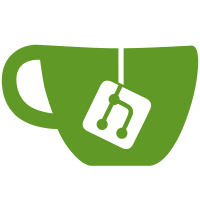
Currently we store all access tokens in the DB, and fall back to that check if we can't validate the macaroon, so our fallback works here, but for guests, their macaroons don't get persisted, so we don't get to find them in the database. Each restart, we generate a new ephemeral key, so guests lose access after each server restart. I tried to fix up the config stuff to be less insane, but gave up, so instead I bolt on yet another piece of custom one-off insanity. Also, add some basic tests for config generation and loading.
94 lines
3.5 KiB
Python
94 lines
3.5 KiB
Python
# -*- coding: utf-8 -*-
|
|
# Copyright 2015, 2016 OpenMarket Ltd
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License");
|
|
# you may not use this file except in compliance with the License.
|
|
# You may obtain a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS,
|
|
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
# See the License for the specific language governing permissions and
|
|
# limitations under the License.
|
|
|
|
from ._base import Config
|
|
|
|
from synapse.util.stringutils import random_string_with_symbols
|
|
|
|
from distutils.util import strtobool
|
|
|
|
|
|
class RegistrationConfig(Config):
|
|
|
|
def read_config(self, config):
|
|
self.enable_registration = bool(
|
|
strtobool(str(config["enable_registration"]))
|
|
)
|
|
if "disable_registration" in config:
|
|
self.enable_registration = not bool(
|
|
strtobool(str(config["disable_registration"]))
|
|
)
|
|
|
|
self.registration_shared_secret = config.get("registration_shared_secret")
|
|
self.macaroon_secret_key = config.get("macaroon_secret_key")
|
|
if self.macaroon_secret_key is None:
|
|
raise Exception(
|
|
"Config is missing missing macaroon_secret_key - please set it"
|
|
" in your config file."
|
|
)
|
|
self.bcrypt_rounds = config.get("bcrypt_rounds", 12)
|
|
self.trusted_third_party_id_servers = config["trusted_third_party_id_servers"]
|
|
self.allow_guest_access = config.get("allow_guest_access", False)
|
|
|
|
def default_config(self, is_generating_file=False, **kwargs):
|
|
registration_shared_secret = random_string_with_symbols(50)
|
|
|
|
macaroon_line = ""
|
|
if is_generating_file:
|
|
macaroon_line += '\n macaroon_secret_key: "%s"\n' % (
|
|
random_string_with_symbols(50),
|
|
)
|
|
|
|
macaroon_secret_key = random_string_with_symbols(50)
|
|
return """\
|
|
## Registration ##
|
|
|
|
# Enable registration for new users.
|
|
enable_registration: False
|
|
|
|
# If set, allows registration by anyone who also has the shared
|
|
# secret, even if registration is otherwise disabled.
|
|
registration_shared_secret: "%(registration_shared_secret)s"
|
|
%(macaroon_line)s
|
|
# Set the number of bcrypt rounds used to generate password hash.
|
|
# Larger numbers increase the work factor needed to generate the hash.
|
|
# The default number of rounds is 12.
|
|
bcrypt_rounds: 12
|
|
|
|
# Allows users to register as guests without a password/email/etc, and
|
|
# participate in rooms hosted on this server which have been made
|
|
# accessible to anonymous users.
|
|
allow_guest_access: False
|
|
|
|
# The list of identity servers trusted to verify third party
|
|
# identifiers by this server.
|
|
trusted_third_party_id_servers:
|
|
- matrix.org
|
|
- vector.im
|
|
""" % locals()
|
|
|
|
def add_arguments(self, parser):
|
|
reg_group = parser.add_argument_group("registration")
|
|
reg_group.add_argument(
|
|
"--enable-registration", action="store_true", default=None,
|
|
help="Enable registration for new users."
|
|
)
|
|
|
|
def read_arguments(self, args):
|
|
if args.enable_registration is not None:
|
|
self.enable_registration = bool(
|
|
strtobool(str(args.enable_registration))
|
|
)
|