mirror of
https://git.anonymousland.org/anonymousland/synapse.git
synced 2025-07-15 16:19:26 -04:00
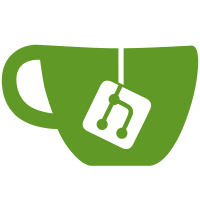
This should help ensure that equivalent results are achieved between homeservers querying for the summary of a space. This implements modified MSC1772 rules, according to MSC2946. The different is that the origin_server_ts of the m.room.create event is not used as a tie-breaker since this might not be known if the homeserver is not part of the room.
81 lines
2.7 KiB
Python
81 lines
2.7 KiB
Python
# Copyright 2021 The Matrix.org Foundation C.I.C.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License");
|
|
# you may not use this file except in compliance with the License.
|
|
# You may obtain a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS,
|
|
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
# See the License for the specific language governing permissions and
|
|
# limitations under the License.
|
|
from typing import Any, Optional
|
|
from unittest import mock
|
|
|
|
from synapse.handlers.space_summary import _child_events_comparison_key
|
|
|
|
from tests import unittest
|
|
|
|
|
|
def _create_event(room_id: str, order: Optional[Any] = None):
|
|
result = mock.Mock()
|
|
result.room_id = room_id
|
|
result.content = {}
|
|
if order is not None:
|
|
result.content["order"] = order
|
|
return result
|
|
|
|
|
|
def _order(*events):
|
|
return sorted(events, key=_child_events_comparison_key)
|
|
|
|
|
|
class TestSpaceSummarySort(unittest.TestCase):
|
|
def test_no_order_last(self):
|
|
"""An event with no ordering is placed behind those with an ordering."""
|
|
ev1 = _create_event("!abc:test")
|
|
ev2 = _create_event("!xyz:test", "xyz")
|
|
|
|
self.assertEqual([ev2, ev1], _order(ev1, ev2))
|
|
|
|
def test_order(self):
|
|
"""The ordering should be used."""
|
|
ev1 = _create_event("!abc:test", "xyz")
|
|
ev2 = _create_event("!xyz:test", "abc")
|
|
|
|
self.assertEqual([ev2, ev1], _order(ev1, ev2))
|
|
|
|
def test_order_room_id(self):
|
|
"""Room ID is a tie-breaker for ordering."""
|
|
ev1 = _create_event("!abc:test", "abc")
|
|
ev2 = _create_event("!xyz:test", "abc")
|
|
|
|
self.assertEqual([ev1, ev2], _order(ev1, ev2))
|
|
|
|
def test_invalid_ordering_type(self):
|
|
"""Invalid orderings are considered the same as missing."""
|
|
ev1 = _create_event("!abc:test", 1)
|
|
ev2 = _create_event("!xyz:test", "xyz")
|
|
|
|
self.assertEqual([ev2, ev1], _order(ev1, ev2))
|
|
|
|
ev1 = _create_event("!abc:test", {})
|
|
self.assertEqual([ev2, ev1], _order(ev1, ev2))
|
|
|
|
ev1 = _create_event("!abc:test", [])
|
|
self.assertEqual([ev2, ev1], _order(ev1, ev2))
|
|
|
|
ev1 = _create_event("!abc:test", True)
|
|
self.assertEqual([ev2, ev1], _order(ev1, ev2))
|
|
|
|
def test_invalid_ordering_value(self):
|
|
"""Invalid orderings are considered the same as missing."""
|
|
ev1 = _create_event("!abc:test", "foo\n")
|
|
ev2 = _create_event("!xyz:test", "xyz")
|
|
|
|
self.assertEqual([ev2, ev1], _order(ev1, ev2))
|
|
|
|
ev1 = _create_event("!abc:test", "a" * 51)
|
|
self.assertEqual([ev2, ev1], _order(ev1, ev2))
|