mirror of
https://github.com/PrivateBin/PrivateBin.git
synced 2025-07-03 02:46:43 -04:00
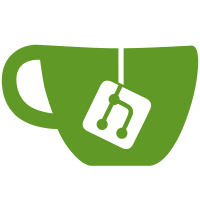
- addresses #1365 - should make upgrades easier for those using custom templates - if the JS files got customized, the default SRI hashes can be replaced in the conf.php file, added commented section in conf.sample.php
78 lines
2 KiB
PHP
78 lines
2 KiB
PHP
<?php declare(strict_types=1);
|
|
/**
|
|
* PrivateBin
|
|
*
|
|
* a zero-knowledge paste bin
|
|
*
|
|
* @link https://github.com/PrivateBin/PrivateBin
|
|
* @copyright 2012 Sébastien SAUVAGE (sebsauvage.net)
|
|
* @license https://www.opensource.org/licenses/zlib-license.php The zlib/libpng License
|
|
*/
|
|
|
|
namespace PrivateBin;
|
|
|
|
use Exception;
|
|
|
|
/**
|
|
* View
|
|
*
|
|
* Displays the templates
|
|
*/
|
|
class View
|
|
{
|
|
/**
|
|
* variables available in the template
|
|
*
|
|
* @access private
|
|
* @var array
|
|
*/
|
|
private $_variables = array();
|
|
|
|
/**
|
|
* assign variables to be used inside of the template
|
|
*
|
|
* @access public
|
|
* @param string $name
|
|
* @param mixed $value
|
|
*/
|
|
public function assign($name, $value)
|
|
{
|
|
$this->_variables[$name] = $value;
|
|
}
|
|
|
|
/**
|
|
* render a template
|
|
*
|
|
* @access public
|
|
* @param string $template
|
|
* @throws Exception
|
|
*/
|
|
public function draw($template)
|
|
{
|
|
$file = substr($template, 0, 10) === 'bootstrap-' ? 'bootstrap' : $template;
|
|
$path = PATH . 'tpl' . DIRECTORY_SEPARATOR . $file . '.php';
|
|
if (!file_exists($path)) {
|
|
throw new Exception('Template ' . $template . ' not found!', 80);
|
|
}
|
|
extract($this->_variables);
|
|
include $path;
|
|
}
|
|
|
|
/**
|
|
* echo script tag incl. SRI hash for given script file
|
|
*
|
|
* @access private
|
|
* @param string $file
|
|
* @param bool $async should it execute ASAP or only after HTML got parsed
|
|
*/
|
|
private function _scriptTag($file, $async = true)
|
|
{
|
|
$sri = array_key_exists($file, $this->_variables['SRI']) ?
|
|
' integrity="' . $this->_variables['SRI'][$file] . '"' : '';
|
|
$suffix = preg_match('#\d.js$#', $file) == 0 ?
|
|
'?' . rawurlencode($this->_variables['VERSION']) : '';
|
|
echo '<script ', $async ? 'async' : 'defer',
|
|
' type="text/javascript" data-cfasync="false" src="', $file,
|
|
$suffix, '"', $sri, ' crossorigin="anonymous"></script>', PHP_EOL;
|
|
}
|
|
}
|