mirror of
https://github.com/BookStackApp/BookStack.git
synced 2024-10-01 01:36:00 -04:00
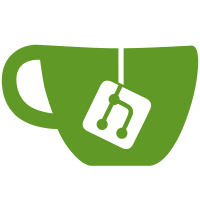
- Fixed issue where redirect for `/settings` view would not be ran through base url generator so would not create a correct path in some cases. Now routed through controller with normal redirect. - Fixed custom head content being active on settings pages due to route name changes, for when viewing settings, in last release. Fixes #3356 and #3355
44 lines
1.2 KiB
PHP
44 lines
1.2 KiB
PHP
<?php
|
|
|
|
namespace Tests\Settings;
|
|
|
|
use Tests\TestCase;
|
|
|
|
class SettingsTest extends TestCase
|
|
{
|
|
public function test_settings_endpoint_redirects_to_settings_view()
|
|
{
|
|
$resp = $this->asAdmin()->get('/settings');
|
|
|
|
$resp->assertStatus(302);
|
|
|
|
// Manually check path to ensure it's generated as the full path
|
|
$location = $resp->headers->get('location');
|
|
$this->assertEquals(url('/settings/features'), $location);
|
|
}
|
|
|
|
public function test_settings_category_links_work_as_expected()
|
|
{
|
|
$this->asAdmin();
|
|
$categories = [
|
|
'features' => 'Features & Security',
|
|
'customization' => 'Customization',
|
|
'registration' => 'Registration',
|
|
];
|
|
|
|
foreach ($categories as $category => $title) {
|
|
$resp = $this->get("/settings/{$category}");
|
|
$resp->assertElementContains('h1', $title);
|
|
$resp->assertElementExists("form[action$=\"/settings/{$category}\"]");
|
|
}
|
|
}
|
|
|
|
public function test_not_found_setting_category_throws_404()
|
|
{
|
|
$resp = $this->asAdmin()->get('/settings/biscuits');
|
|
|
|
$resp->assertStatus(404);
|
|
$resp->assertSee('Page Not Found');
|
|
}
|
|
}
|