mirror of
https://github.com/BookStackApp/BookStack.git
synced 2024-10-01 01:36:00 -04:00
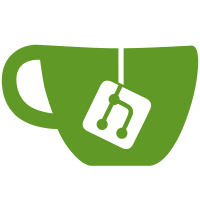
Added test and changed logic to properly check the view permissions for the notification receiver before sending. Required change to permissions applicator to allow the user to be manually determined, and a service provider update to provide the class as a singleton without a specific user, so it checks the current logged in user on demand.
43 lines
1.4 KiB
PHP
43 lines
1.4 KiB
PHP
<?php
|
|
|
|
namespace BookStack\Activity\Notifications\Handlers;
|
|
|
|
use BookStack\Activity\Models\Loggable;
|
|
use BookStack\Activity\Notifications\Messages\BaseActivityNotification;
|
|
use BookStack\Entities\Models\Entity;
|
|
use BookStack\Permissions\PermissionApplicator;
|
|
use BookStack\Users\Models\User;
|
|
|
|
abstract class BaseNotificationHandler implements NotificationHandler
|
|
{
|
|
/**
|
|
* @param class-string<BaseActivityNotification> $notification
|
|
* @param int[] $userIds
|
|
*/
|
|
protected function sendNotificationToUserIds(string $notification, array $userIds, User $initiator, string|Loggable $detail, Entity $relatedModel): void
|
|
{
|
|
$users = User::query()->whereIn('id', array_unique($userIds))->get();
|
|
|
|
foreach ($users as $user) {
|
|
// Prevent sending to the user that initiated the activity
|
|
if ($user->id === $initiator->id) {
|
|
continue;
|
|
}
|
|
|
|
// Prevent sending of the user does not have notification permissions
|
|
if (!$user->can('receive-notifications')) {
|
|
continue;
|
|
}
|
|
|
|
// Prevent sending if the user does not have access to the related content
|
|
$permissions = new PermissionApplicator($user);
|
|
if (!$permissions->checkOwnableUserAccess($relatedModel, 'view')) {
|
|
continue;
|
|
}
|
|
|
|
// Send the notification
|
|
$user->notify(new $notification($detail, $initiator));
|
|
}
|
|
}
|
|
}
|