mirror of
https://github.com/BookStackApp/BookStack.git
synced 2024-07-02 17:41:45 +00:00
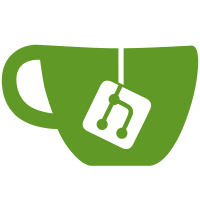
Fixes issue where certain errors would not show to the user due to extra navigation jumps which lost the error message in the process. This simplifies and aligns exceptions with more directly handled exception usage at the controller level. Fixes #3264
51 lines
1.2 KiB
PHP
51 lines
1.2 KiB
PHP
<?php
|
|
|
|
namespace BookStack\Exceptions;
|
|
|
|
use Exception;
|
|
use Illuminate\Contracts\Support\Responsable;
|
|
|
|
class NotifyException extends Exception implements Responsable
|
|
{
|
|
public $message;
|
|
public $redirectLocation;
|
|
protected $status;
|
|
|
|
public function __construct(string $message, string $redirectLocation = '/', int $status = 500)
|
|
{
|
|
$this->message = $message;
|
|
$this->redirectLocation = $redirectLocation;
|
|
$this->status = $status;
|
|
parent::__construct();
|
|
}
|
|
|
|
/**
|
|
* Get the desired status code for this exception.
|
|
*/
|
|
public function getStatus(): int
|
|
{
|
|
return $this->status;
|
|
}
|
|
|
|
/**
|
|
* Send the response for this type of exception.
|
|
*
|
|
* {@inheritdoc}
|
|
*/
|
|
public function toResponse($request)
|
|
{
|
|
$message = $this->getMessage();
|
|
|
|
// Front-end JSON handling. API-side handling managed via handler.
|
|
if ($request->wantsJson()) {
|
|
return response()->json(['error' => $message], 403);
|
|
}
|
|
|
|
if (!empty($message)) {
|
|
session()->flash('error', $message);
|
|
}
|
|
|
|
return redirect($this->redirectLocation);
|
|
}
|
|
}
|