mirror of
https://github.com/BookStackApp/BookStack.git
synced 2024-10-01 01:36:00 -04:00
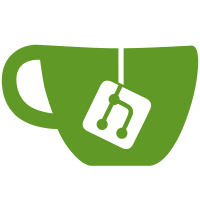
Also implemented more elegant solution to allowing session auth for API routes; A new 'StartSessionIfCookieExists' middleware, which wraps the default 'StartSession' middleware will run for API routes which only sets up the session if a session cookie is found on the request. Also decrypts only the session cookie. Also cleaned some TokenController codeclimate warnings.
32 lines
726 B
PHP
32 lines
726 B
PHP
<?php namespace BookStack\Api;
|
|
|
|
use BookStack\Auth\User;
|
|
use Illuminate\Database\Eloquent\Model;
|
|
use Illuminate\Database\Eloquent\Relations\BelongsTo;
|
|
use Illuminate\Support\Carbon;
|
|
|
|
class ApiToken extends Model
|
|
{
|
|
protected $fillable = ['name', 'expires_at'];
|
|
protected $casts = [
|
|
'expires_at' => 'date:Y-m-d'
|
|
];
|
|
|
|
/**
|
|
* Get the user that this token belongs to.
|
|
*/
|
|
public function user(): BelongsTo
|
|
{
|
|
return $this->belongsTo(User::class);
|
|
}
|
|
|
|
/**
|
|
* Get the default expiry value for an API token.
|
|
* Set to 100 years from now.
|
|
*/
|
|
public static function defaultExpiry(): string
|
|
{
|
|
return Carbon::now()->addYears(100)->format('Y-m-d');
|
|
}
|
|
}
|