mirror of
https://github.com/BookStackApp/BookStack.git
synced 2024-08-26 12:16:07 +00:00
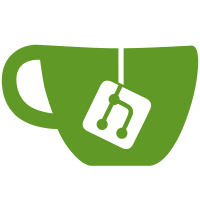
Failure of loading drawings will now close the drawing view and show an error message, hinting at file or permission issues, instead of leaving the user facing a continuosly loading interface. Adds test to cover. This also updates errors from our HTTP service to be wrapped in a custom error type for better identification and so the error is an actual javascript error. Should be object compatible. Related to #3955.
76 lines
1.9 KiB
JavaScript
76 lines
1.9 KiB
JavaScript
const listeners = {};
|
|
const stack = [];
|
|
|
|
/**
|
|
* Emit a custom event for any handlers to pick-up.
|
|
* @param {String} eventName
|
|
* @param {*} eventData
|
|
*/
|
|
function emit(eventName, eventData) {
|
|
stack.push({name: eventName, data: eventData});
|
|
if (typeof listeners[eventName] === 'undefined') return this;
|
|
let eventsToStart = listeners[eventName];
|
|
for (let i = 0; i < eventsToStart.length; i++) {
|
|
let event = eventsToStart[i];
|
|
event(eventData);
|
|
}
|
|
}
|
|
|
|
/**
|
|
* Listen to a custom event and run the given callback when that event occurs.
|
|
* @param {String} eventName
|
|
* @param {Function} callback
|
|
* @returns {Events}
|
|
*/
|
|
function listen(eventName, callback) {
|
|
if (typeof listeners[eventName] === 'undefined') listeners[eventName] = [];
|
|
listeners[eventName].push(callback);
|
|
}
|
|
|
|
/**
|
|
* Emit an event for public use.
|
|
* Sends the event via the native DOM event handling system.
|
|
* @param {Element} targetElement
|
|
* @param {String} eventName
|
|
* @param {Object} eventData
|
|
*/
|
|
function emitPublic(targetElement, eventName, eventData) {
|
|
const event = new CustomEvent(eventName, {
|
|
detail: eventData,
|
|
bubbles: true
|
|
});
|
|
targetElement.dispatchEvent(event);
|
|
}
|
|
|
|
/**
|
|
* Notify of standard server-provided validation errors.
|
|
* @param {Object} error
|
|
*/
|
|
function showValidationErrors(error) {
|
|
if (!error.status) return;
|
|
if (error.status === 422 && error.data) {
|
|
const message = Object.values(error.data).flat().join('\n');
|
|
emit('error', message);
|
|
}
|
|
}
|
|
|
|
/**
|
|
* Notify standard server-provided error messages.
|
|
* @param {Object} error
|
|
*/
|
|
function showResponseError(error) {
|
|
if (!error.status) return;
|
|
if (error.status >= 400 && error.data && error.data.message) {
|
|
emit('error', error.data.message);
|
|
}
|
|
}
|
|
|
|
export default {
|
|
emit,
|
|
emitPublic,
|
|
listen,
|
|
success: (msg) => emit('success', msg),
|
|
error: (msg) => emit('error', msg),
|
|
showValidationErrors,
|
|
showResponseError,
|
|
} |