mirror of
https://github.com/BookStackApp/BookStack.git
synced 2024-07-17 16:52:24 +00:00
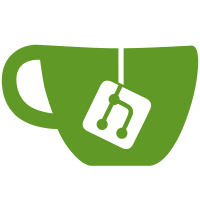
Page-related items added on drafts could be visible in certain scenarios since the applied permissions query filters would not consider page draft visibility. This commit alters queries on related items to apply such filtering. Included test to cover API scenario. Thanks to @haxatron for reporting.
139 lines
3.4 KiB
PHP
139 lines
3.4 KiB
PHP
<?php
|
|
|
|
namespace BookStack\Exceptions;
|
|
|
|
use Exception;
|
|
use Illuminate\Auth\AuthenticationException;
|
|
use Illuminate\Database\Eloquent\ModelNotFoundException;
|
|
use Illuminate\Foundation\Exceptions\Handler as ExceptionHandler;
|
|
use Illuminate\Http\JsonResponse;
|
|
use Illuminate\Http\Request;
|
|
use Illuminate\Validation\ValidationException;
|
|
use Symfony\Component\HttpKernel\Exception\HttpException;
|
|
use Throwable;
|
|
|
|
class Handler extends ExceptionHandler
|
|
{
|
|
/**
|
|
* A list of the exception types that are not reported.
|
|
*
|
|
* @var array
|
|
*/
|
|
protected $dontReport = [
|
|
NotFoundException::class,
|
|
];
|
|
|
|
/**
|
|
* A list of the inputs that are never flashed for validation exceptions.
|
|
*
|
|
* @var array
|
|
*/
|
|
protected $dontFlash = [
|
|
'current_password',
|
|
'password',
|
|
'password_confirmation',
|
|
];
|
|
|
|
/**
|
|
* Report or log an exception.
|
|
*
|
|
* @param \Throwable $exception
|
|
*
|
|
* @throws \Throwable
|
|
*
|
|
* @return void
|
|
*/
|
|
public function report(Throwable $exception)
|
|
{
|
|
parent::report($exception);
|
|
}
|
|
|
|
/**
|
|
* Render an exception into an HTTP response.
|
|
*
|
|
* @param \Illuminate\Http\Request $request
|
|
* @param Exception $e
|
|
*
|
|
* @return \Illuminate\Http\Response
|
|
*/
|
|
public function render($request, Throwable $e)
|
|
{
|
|
if ($this->isApiRequest($request)) {
|
|
return $this->renderApiException($e);
|
|
}
|
|
|
|
return parent::render($request, $e);
|
|
}
|
|
|
|
/**
|
|
* Check if the given request is an API request.
|
|
*/
|
|
protected function isApiRequest(Request $request): bool
|
|
{
|
|
return strpos($request->path(), 'api/') === 0;
|
|
}
|
|
|
|
/**
|
|
* Render an exception when the API is in use.
|
|
*/
|
|
protected function renderApiException(Throwable $e): JsonResponse
|
|
{
|
|
$code = 500;
|
|
$headers = [];
|
|
|
|
if ($e instanceof HttpException) {
|
|
$code = $e->getStatusCode();
|
|
$headers = $e->getHeaders();
|
|
}
|
|
|
|
if ($e instanceof ModelNotFoundException) {
|
|
$code = 404;
|
|
}
|
|
|
|
$responseData = [
|
|
'error' => [
|
|
'message' => $e->getMessage(),
|
|
],
|
|
];
|
|
|
|
if ($e instanceof ValidationException) {
|
|
$responseData['error']['validation'] = $e->errors();
|
|
$code = $e->status;
|
|
}
|
|
|
|
$responseData['error']['code'] = $code;
|
|
|
|
return new JsonResponse($responseData, $code, $headers);
|
|
}
|
|
|
|
/**
|
|
* Convert an authentication exception into an unauthenticated response.
|
|
*
|
|
* @param \Illuminate\Http\Request $request
|
|
* @param \Illuminate\Auth\AuthenticationException $exception
|
|
*
|
|
* @return \Illuminate\Http\Response
|
|
*/
|
|
protected function unauthenticated($request, AuthenticationException $exception)
|
|
{
|
|
if ($request->expectsJson()) {
|
|
return response()->json(['error' => 'Unauthenticated.'], 401);
|
|
}
|
|
|
|
return redirect()->guest('login');
|
|
}
|
|
|
|
/**
|
|
* Convert a validation exception into a JSON response.
|
|
*
|
|
* @param \Illuminate\Http\Request $request
|
|
* @param \Illuminate\Validation\ValidationException $exception
|
|
*
|
|
* @return \Illuminate\Http\JsonResponse
|
|
*/
|
|
protected function invalidJson($request, ValidationException $exception)
|
|
{
|
|
return response()->json($exception->errors(), $exception->status);
|
|
}
|
|
}
|