mirror of
https://github.com/BookStackApp/BookStack.git
synced 2024-10-01 01:36:00 -04:00
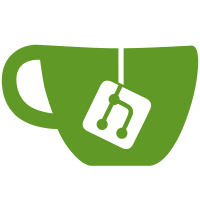
Kept existing "COMMENTED_ON" activity for upgrade compatibility, specifically for existing webhook usage and for showing comment activities in activity lists. Precursor to content notifications. Currently untested. Also applied some type updates.
68 lines
1.5 KiB
PHP
68 lines
1.5 KiB
PHP
<?php
|
|
|
|
namespace BookStack\Activity\Models;
|
|
|
|
use BookStack\App\Model;
|
|
use BookStack\Users\Models\HasCreatorAndUpdater;
|
|
use Illuminate\Database\Eloquent\Factories\HasFactory;
|
|
use Illuminate\Database\Eloquent\Relations\MorphTo;
|
|
|
|
/**
|
|
* @property int $id
|
|
* @property string $text
|
|
* @property string $html
|
|
* @property int|null $parent_id
|
|
* @property int $local_id
|
|
* @property string $entity_type
|
|
* @property int $entity_id
|
|
*/
|
|
class Comment extends Model implements Loggable
|
|
{
|
|
use HasFactory;
|
|
use HasCreatorAndUpdater;
|
|
|
|
protected $fillable = ['text', 'parent_id'];
|
|
protected $appends = ['created', 'updated'];
|
|
|
|
/**
|
|
* Get the entity that this comment belongs to.
|
|
*/
|
|
public function entity(): MorphTo
|
|
{
|
|
return $this->morphTo('entity');
|
|
}
|
|
|
|
/**
|
|
* Check if a comment has been updated since creation.
|
|
*/
|
|
public function isUpdated(): bool
|
|
{
|
|
return $this->updated_at->timestamp > $this->created_at->timestamp;
|
|
}
|
|
|
|
/**
|
|
* Get created date as a relative diff.
|
|
*
|
|
* @return mixed
|
|
*/
|
|
public function getCreatedAttribute()
|
|
{
|
|
return $this->created_at->diffForHumans();
|
|
}
|
|
|
|
/**
|
|
* Get updated date as a relative diff.
|
|
*
|
|
* @return mixed
|
|
*/
|
|
public function getUpdatedAttribute()
|
|
{
|
|
return $this->updated_at->diffForHumans();
|
|
}
|
|
|
|
public function logDescriptor(): string
|
|
{
|
|
return "Comment #{$this->local_id} (ID: {$this->id}) for {$this->entity_type} (ID: {$this->entity_id})";
|
|
}
|
|
}
|