mirror of
https://github.com/BookStackApp/BookStack.git
synced 2024-08-28 21:22:30 +00:00
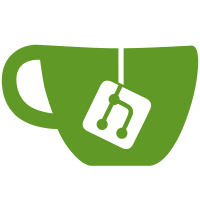
Models are now loaded into their own map to then be used for sorting and reporting back of changed books. Prevents akward logic ordering issues of before where some bits of code assumed/hoped for loaded models on abstract data structures. New levels of permissions are now checked for items within the sort operation. Needs testing to cover.
73 lines
2.1 KiB
PHP
73 lines
2.1 KiB
PHP
<?php
|
|
|
|
namespace BookStack\Http\Controllers;
|
|
|
|
use BookStack\Actions\ActivityType;
|
|
use BookStack\Entities\Repos\BookRepo;
|
|
use BookStack\Entities\Tools\BookContents;
|
|
use BookStack\Entities\Tools\BookSortMap;
|
|
use BookStack\Facades\Activity;
|
|
use Illuminate\Http\Request;
|
|
|
|
class BookSortController extends Controller
|
|
{
|
|
protected $bookRepo;
|
|
|
|
public function __construct(BookRepo $bookRepo)
|
|
{
|
|
$this->bookRepo = $bookRepo;
|
|
}
|
|
|
|
/**
|
|
* Shows the view which allows pages to be re-ordered and sorted.
|
|
*/
|
|
public function show(string $bookSlug)
|
|
{
|
|
$book = $this->bookRepo->getBySlug($bookSlug);
|
|
$this->checkOwnablePermission('book-update', $book);
|
|
|
|
$bookChildren = (new BookContents($book))->getTree(false);
|
|
|
|
$this->setPageTitle(trans('entities.books_sort_named', ['bookName'=>$book->getShortName()]));
|
|
|
|
return view('books.sort', ['book' => $book, 'current' => $book, 'bookChildren' => $bookChildren]);
|
|
}
|
|
|
|
/**
|
|
* Shows the sort box for a single book.
|
|
* Used via AJAX when loading in extra books to a sort.
|
|
*/
|
|
public function showItem(string $bookSlug)
|
|
{
|
|
$book = $this->bookRepo->getBySlug($bookSlug);
|
|
$bookChildren = (new BookContents($book))->getTree();
|
|
|
|
return view('books.parts.sort-box', ['book' => $book, 'bookChildren' => $bookChildren]);
|
|
}
|
|
|
|
/**
|
|
* Sorts a book using a given mapping array.
|
|
*/
|
|
public function update(Request $request, string $bookSlug)
|
|
{
|
|
$book = $this->bookRepo->getBySlug($bookSlug);
|
|
$this->checkOwnablePermission('book-update', $book);
|
|
|
|
// Return if no map sent
|
|
if (!$request->filled('sort-tree')) {
|
|
return redirect($book->getUrl());
|
|
}
|
|
|
|
$sortMap = BookSortMap::fromJson($request->get('sort-tree'));
|
|
$bookContents = new BookContents($book);
|
|
$booksInvolved = $bookContents->sortUsingMap($sortMap);
|
|
|
|
// Rebuild permissions and add activity for involved books.
|
|
foreach ($booksInvolved as $bookInvolved) {
|
|
Activity::add(ActivityType::BOOK_SORT, $bookInvolved);
|
|
}
|
|
|
|
return redirect($book->getUrl());
|
|
}
|
|
}
|