mirror of
https://github.com/BookStackApp/BookStack.git
synced 2024-07-17 00:32:36 +00:00
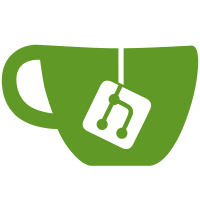
- Updates old components to newer format, removes legacy component support. - Makes component registration easier and less duplicated. - Adds base component class to extend for better editor support. - Aligns global window exposure usage and aligns with other service names.
58 lines
1.4 KiB
JavaScript
58 lines
1.4 KiB
JavaScript
export class Component {
|
|
|
|
/**
|
|
* The registered name of the component.
|
|
* @type {string}
|
|
*/
|
|
$name = '';
|
|
|
|
/**
|
|
* The element that the component is registered upon.
|
|
* @type {Element}
|
|
*/
|
|
$el = null;
|
|
|
|
/**
|
|
* Mapping of referenced elements within the component.
|
|
* @type {Object<string, Element>}
|
|
*/
|
|
$refs = {};
|
|
|
|
/**
|
|
* Mapping of arrays of referenced elements within the component so multiple
|
|
* references, sharing the same name, can be fetched.
|
|
* @type {Object<string, Element[]>}
|
|
*/
|
|
$manyRefs = {};
|
|
|
|
/**
|
|
* Options passed into this component.
|
|
* @type {Object<String, String>}
|
|
*/
|
|
$opts = {};
|
|
|
|
/**
|
|
* Component-specific setup methods.
|
|
* Use this to assign local variables and run any initial setup or actions.
|
|
*/
|
|
setup() {
|
|
//
|
|
}
|
|
|
|
/**
|
|
* Emit an event from this component.
|
|
* Will be bubbled up from the dom element this is registered on, as a custom event
|
|
* with the name `<elementName>-<eventName>`, with the provided data in the event detail.
|
|
* @param {String} eventName
|
|
* @param {Object} data
|
|
*/
|
|
$emit(eventName, data = {}) {
|
|
data.from = this;
|
|
const componentName = this.$name;
|
|
const event = new CustomEvent(`${componentName}-${eventName}`, {
|
|
bubbles: true,
|
|
detail: data
|
|
});
|
|
this.$el.dispatchEvent(event);
|
|
}
|
|
} |