mirror of
https://github.com/BookStackApp/BookStack.git
synced 2024-10-01 01:36:00 -04:00
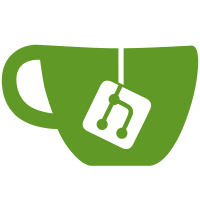
* Temporarily moved back config path * Apply Laravel coding style * Shift exception handler * Shift HTTP kernel and middleware * Shift service providers * Convert options array to fluent methods * Shift to class based routes * Shift console routes * Ignore temporary framework files * Shift to class based factories * Namespace seeders * Shift PSR-4 autoloading * Shift config files * Default config files * Shift Laravel dependencies * Shift return type of base TestCase methods * Shift cleanup * Applied stylci style changes * Reverted config files location * Applied manual changes to Laravel 8 shift Co-authored-by: Shift <shift@laravelshift.com>
47 lines
1.1 KiB
PHP
47 lines
1.1 KiB
PHP
<?php
|
|
|
|
namespace BookStack\Uploads;
|
|
|
|
use BookStack\Entities\Models\Page;
|
|
use BookStack\Model;
|
|
use BookStack\Traits\HasCreatorAndUpdater;
|
|
use Illuminate\Database\Eloquent\Factories\HasFactory;
|
|
|
|
/**
|
|
* @property int $id
|
|
* @property string $name
|
|
* @property string $url
|
|
* @property string $path
|
|
* @property string $type
|
|
* @property int $uploaded_to
|
|
* @property int $created_by
|
|
* @property int $updated_by
|
|
*/
|
|
class Image extends Model
|
|
{
|
|
use HasFactory;
|
|
use HasCreatorAndUpdater;
|
|
|
|
protected $fillable = ['name'];
|
|
protected $hidden = [];
|
|
|
|
/**
|
|
* Get a thumbnail for this image.
|
|
*
|
|
* @throws \Exception
|
|
*/
|
|
public function getThumb(int $width, int $height, bool $keepRatio = false): string
|
|
{
|
|
return app()->make(ImageService::class)->getThumbnail($this, $width, $height, $keepRatio);
|
|
}
|
|
|
|
/**
|
|
* Get the page this image has been uploaded to.
|
|
* Only applicable to gallery or drawio image types.
|
|
*/
|
|
public function getPage(): ?Page
|
|
{
|
|
return $this->belongsTo(Page::class, 'uploaded_to')->first();
|
|
}
|
|
}
|