mirror of
https://github.com/BookStackApp/BookStack.git
synced 2024-10-01 01:36:00 -04:00
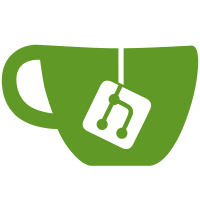
* Temporarily moved back config path * Apply Laravel coding style * Shift exception handler * Shift HTTP kernel and middleware * Shift service providers * Convert options array to fluent methods * Shift to class based routes * Shift console routes * Ignore temporary framework files * Shift to class based factories * Namespace seeders * Shift PSR-4 autoloading * Shift config files * Default config files * Shift Laravel dependencies * Shift return type of base TestCase methods * Shift cleanup * Applied stylci style changes * Reverted config files location * Applied manual changes to Laravel 8 shift Co-authored-by: Shift <shift@laravelshift.com>
92 lines
2.2 KiB
PHP
92 lines
2.2 KiB
PHP
<?php
|
|
|
|
namespace BookStack\Providers;
|
|
|
|
use Illuminate\Cache\RateLimiting\Limit;
|
|
use Illuminate\Foundation\Support\Providers\RouteServiceProvider as ServiceProvider;
|
|
use Illuminate\Http\Request;
|
|
use Illuminate\Support\Facades\RateLimiter;
|
|
use Illuminate\Support\Facades\Route;
|
|
|
|
class RouteServiceProvider extends ServiceProvider
|
|
{
|
|
/**
|
|
* The path to the "home" route for your application.
|
|
*
|
|
* This is used by Laravel authentication to redirect users after login.
|
|
*
|
|
* @var string
|
|
*/
|
|
public const HOME = '/';
|
|
|
|
/**
|
|
* This namespace is applied to the controller routes in your routes file.
|
|
*
|
|
* In addition, it is set as the URL generator's root namespace.
|
|
*
|
|
* @var string
|
|
*/
|
|
|
|
/**
|
|
* Define your route model bindings, pattern filters, etc.
|
|
*
|
|
* @return void
|
|
*/
|
|
public function boot()
|
|
{
|
|
$this->configureRateLimiting();
|
|
|
|
$this->routes(function () {
|
|
$this->mapWebRoutes();
|
|
$this->mapApiRoutes();
|
|
});
|
|
}
|
|
|
|
/**
|
|
* Define the "web" routes for the application.
|
|
*
|
|
* These routes all receive session state, CSRF protection, etc.
|
|
*
|
|
* @return void
|
|
*/
|
|
protected function mapWebRoutes()
|
|
{
|
|
Route::group([
|
|
'middleware' => 'web',
|
|
'namespace' => $this->namespace,
|
|
], function ($router) {
|
|
require base_path('routes/web.php');
|
|
});
|
|
}
|
|
|
|
/**
|
|
* Define the "api" routes for the application.
|
|
*
|
|
* These routes are typically stateless.
|
|
*
|
|
* @return void
|
|
*/
|
|
protected function mapApiRoutes()
|
|
{
|
|
Route::group([
|
|
'middleware' => 'api',
|
|
'namespace' => $this->namespace . '\Api',
|
|
'prefix' => 'api',
|
|
], function ($router) {
|
|
require base_path('routes/api.php');
|
|
});
|
|
}
|
|
|
|
/**
|
|
* Configure the rate limiters for the application.
|
|
*
|
|
* @return void
|
|
*/
|
|
protected function configureRateLimiting()
|
|
{
|
|
RateLimiter::for('api', function (Request $request) {
|
|
return Limit::perMinute(60)->by(optional($request->user())->id ?: $request->ip());
|
|
});
|
|
}
|
|
}
|