mirror of
https://github.com/BookStackApp/BookStack.git
synced 2024-10-01 01:36:00 -04:00
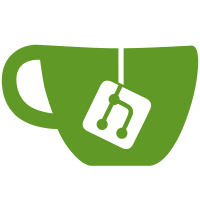
* Started mass-refactoring of the current entity repos * Rewrote book tree logic - Now does two simple queries instead of one really complex one. - Extracted logic into its own class. - Remove model-level akward union field listing. - Logic now more readable than being large separate query and compilation functions. * Extracted and split book sort logic * Finished up Book controller/repo organisation * Refactored bookshelves controllers and repo parts * Fixed issues found via phpunit * Refactored Chapter controller * Updated Chapter export controller * Started Page controller/repo refactor * Refactored another chunk of PageController * Completed initial pagecontroller refactor pass * Fixed tests and continued reduction of old repos * Removed old page remove and further reduced entity repo * Removed old entity repo, split out page controller * Ran phpcbf and split out some page content methods * Tidied up some EntityProvider elements * Fixed issued caused by viewservice change
93 lines
1.9 KiB
PHP
93 lines
1.9 KiB
PHP
<?php namespace BookStack\Entities;
|
|
|
|
/**
|
|
* Class EntityProvider
|
|
*
|
|
* Provides access to the core entity models.
|
|
* Wrapped up in this provider since they are often used together
|
|
* so this is a neater alternative to injecting all in individually.
|
|
*
|
|
* @package BookStack\Entities
|
|
*/
|
|
class EntityProvider
|
|
{
|
|
|
|
/**
|
|
* @var Bookshelf
|
|
*/
|
|
public $bookshelf;
|
|
|
|
/**
|
|
* @var Book
|
|
*/
|
|
public $book;
|
|
|
|
/**
|
|
* @var Chapter
|
|
*/
|
|
public $chapter;
|
|
|
|
/**
|
|
* @var Page
|
|
*/
|
|
public $page;
|
|
|
|
/**
|
|
* @var PageRevision
|
|
*/
|
|
public $pageRevision;
|
|
|
|
/**
|
|
* EntityProvider constructor.
|
|
*/
|
|
public function __construct(
|
|
Bookshelf $bookshelf,
|
|
Book $book,
|
|
Chapter $chapter,
|
|
Page $page,
|
|
PageRevision $pageRevision
|
|
) {
|
|
$this->bookshelf = $bookshelf;
|
|
$this->book = $book;
|
|
$this->chapter = $chapter;
|
|
$this->page = $page;
|
|
$this->pageRevision = $pageRevision;
|
|
}
|
|
|
|
/**
|
|
* Fetch all core entity types as an associated array
|
|
* with their basic names as the keys.
|
|
*/
|
|
public function all(): array
|
|
{
|
|
return [
|
|
'bookshelf' => $this->bookshelf,
|
|
'book' => $this->book,
|
|
'chapter' => $this->chapter,
|
|
'page' => $this->page,
|
|
];
|
|
}
|
|
|
|
/**
|
|
* Get an entity instance by it's basic name.
|
|
*/
|
|
public function get(string $type): Entity
|
|
{
|
|
$type = strtolower($type);
|
|
return $this->all()[$type];
|
|
}
|
|
|
|
/**
|
|
* Get the morph classes, as an array, for a single or multiple types.
|
|
*/
|
|
public function getMorphClasses(array $types): array
|
|
{
|
|
$morphClasses = [];
|
|
foreach ($types as $type) {
|
|
$model = $this->get($type);
|
|
$morphClasses[] = $model->getMorphClass();
|
|
}
|
|
return $morphClasses;
|
|
}
|
|
}
|