mirror of
https://github.com/BookStackApp/BookStack.git
synced 2024-07-17 08:42:23 +00:00
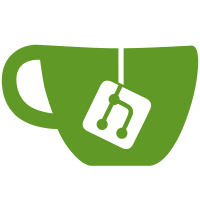
- Removed old 'exposeTranslations' system to instead use new component option system. - Extracted validation rules into their own service provider. - Cleaned up some formatting/comments in the repos.
34 lines
1.1 KiB
PHP
34 lines
1.1 KiB
PHP
<?php
|
|
|
|
namespace BookStack\Providers;
|
|
|
|
use Illuminate\Support\Facades\Validator;
|
|
use Illuminate\Support\ServiceProvider;
|
|
|
|
class CustomValidationServiceProvider extends ServiceProvider
|
|
{
|
|
|
|
/**
|
|
* Register our custom validation rules when the application boots.
|
|
*/
|
|
public function boot(): void
|
|
{
|
|
Validator::extend('image_extension', function ($attribute, $value, $parameters, $validator) {
|
|
$validImageExtensions = ['png', 'jpg', 'jpeg', 'gif', 'webp'];
|
|
return in_array(strtolower($value->getClientOriginalExtension()), $validImageExtensions);
|
|
});
|
|
|
|
Validator::extend('no_double_extension', function ($attribute, $value, $parameters, $validator) {
|
|
$uploadName = $value->getClientOriginalName();
|
|
return substr_count($uploadName, '.') < 2;
|
|
});
|
|
|
|
Validator::extend('safe_url', function ($attribute, $value, $parameters, $validator) {
|
|
$cleanLinkName = strtolower(trim($value));
|
|
$isJs = strpos($cleanLinkName, 'javascript:') === 0;
|
|
$isData = strpos($cleanLinkName, 'data:') === 0;
|
|
return !$isJs && !$isData;
|
|
});
|
|
}
|
|
}
|