mirror of
https://github.com/BookStackApp/BookStack.git
synced 2024-10-01 01:36:00 -04:00
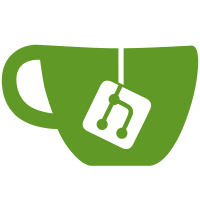
Tools seems to fit better since the classes were a bit of a mixed bunch and did not always manage. Also simplified the structure of the SlugGenerator class. Also focused EntityContext on shelves and simplified to use session helper.
50 lines
1.1 KiB
PHP
50 lines
1.1 KiB
PHP
<?php namespace BookStack\Api;
|
|
|
|
use BookStack\Auth\User;
|
|
use BookStack\Interfaces\Loggable;
|
|
use Illuminate\Database\Eloquent\Model;
|
|
use Illuminate\Database\Eloquent\Relations\BelongsTo;
|
|
use Illuminate\Support\Carbon;
|
|
|
|
/**
|
|
* Class ApiToken
|
|
* @property int $id
|
|
* @property string $token_id
|
|
* @property string $secret
|
|
* @property string $name
|
|
* @property Carbon $expires_at
|
|
* @property User $user
|
|
*/
|
|
class ApiToken extends Model implements Loggable
|
|
{
|
|
protected $fillable = ['name', 'expires_at'];
|
|
protected $casts = [
|
|
'expires_at' => 'date:Y-m-d'
|
|
];
|
|
|
|
/**
|
|
* Get the user that this token belongs to.
|
|
*/
|
|
public function user(): BelongsTo
|
|
{
|
|
return $this->belongsTo(User::class);
|
|
}
|
|
|
|
/**
|
|
* Get the default expiry value for an API token.
|
|
* Set to 100 years from now.
|
|
*/
|
|
public static function defaultExpiry(): string
|
|
{
|
|
return Carbon::now()->addYears(100)->format('Y-m-d');
|
|
}
|
|
|
|
/**
|
|
* @inheritdoc
|
|
*/
|
|
public function logDescriptor(): string
|
|
{
|
|
return "({$this->id}) {$this->name}; User: {$this->user->logDescriptor()}";
|
|
}
|
|
}
|