mirror of
https://github.com/BookStackApp/BookStack.git
synced 2024-09-13 13:04:52 +00:00
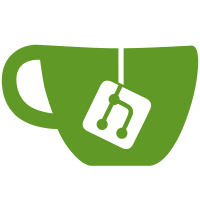
* Started mass-refactoring of the current entity repos * Rewrote book tree logic - Now does two simple queries instead of one really complex one. - Extracted logic into its own class. - Remove model-level akward union field listing. - Logic now more readable than being large separate query and compilation functions. * Extracted and split book sort logic * Finished up Book controller/repo organisation * Refactored bookshelves controllers and repo parts * Fixed issues found via phpunit * Refactored Chapter controller * Updated Chapter export controller * Started Page controller/repo refactor * Refactored another chunk of PageController * Completed initial pagecontroller refactor pass * Fixed tests and continued reduction of old repos * Removed old page remove and further reduced entity repo * Removed old entity repo, split out page controller * Ran phpcbf and split out some page content methods * Tidied up some EntityProvider elements * Fixed issued caused by viewservice change
55 lines
1.3 KiB
PHP
55 lines
1.3 KiB
PHP
<?php namespace BookStack\Entities\Managers;
|
|
|
|
use BookStack\Entities\Book;
|
|
use BookStack\Entities\Bookshelf;
|
|
use Illuminate\Session\Store;
|
|
|
|
class EntityContext
|
|
{
|
|
protected $session;
|
|
|
|
protected $KEY_SHELF_CONTEXT_ID = 'context_bookshelf_id';
|
|
|
|
/**
|
|
* EntityContextManager constructor.
|
|
*/
|
|
public function __construct(Store $session)
|
|
{
|
|
$this->session = $session;
|
|
}
|
|
|
|
/**
|
|
* Get the current bookshelf context for the given book.
|
|
*/
|
|
public function getContextualShelfForBook(Book $book): ?Bookshelf
|
|
{
|
|
$contextBookshelfId = $this->session->get($this->KEY_SHELF_CONTEXT_ID, null);
|
|
|
|
if (!is_int($contextBookshelfId)) {
|
|
return null;
|
|
}
|
|
|
|
$shelf = Bookshelf::visible()->find($contextBookshelfId);
|
|
$shelfContainsBook = $shelf && $shelf->contains($book);
|
|
|
|
return $shelfContainsBook ? $shelf : null;
|
|
}
|
|
|
|
/**
|
|
* Store the current contextual shelf ID.
|
|
* @param int $shelfId
|
|
*/
|
|
public function setShelfContext(int $shelfId)
|
|
{
|
|
$this->session->put($this->KEY_SHELF_CONTEXT_ID, $shelfId);
|
|
}
|
|
|
|
/**
|
|
* Clear the session stored shelf context id.
|
|
*/
|
|
public function clearShelfContext()
|
|
{
|
|
$this->session->forget($this->KEY_SHELF_CONTEXT_ID);
|
|
}
|
|
}
|