mirror of
https://github.com/BookStackApp/BookStack.git
synced 2024-10-01 01:36:00 -04:00
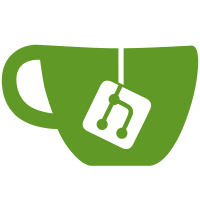
There was a lot of locale handling to get correct/expected date formatting within the app. Carbon now has built-in locale content rather than us needing to target specific system locales. This also removes setting locale via Carbon directly. Carbon registers its own Laravel service provider which seems to accurately pull the correct locale from the app. For #4555
120 lines
3.3 KiB
PHP
120 lines
3.3 KiB
PHP
<?php
|
|
|
|
namespace BookStack\Translation;
|
|
|
|
use BookStack\Users\Models\User;
|
|
use Illuminate\Http\Request;
|
|
|
|
class LocaleManager
|
|
{
|
|
/**
|
|
* Array of right-to-left locale options.
|
|
*/
|
|
protected array $rtlLocales = ['ar', 'fa', 'he'];
|
|
|
|
/**
|
|
* Map of BookStack locale names to best-estimate ISO locale names.
|
|
* Locales can often be found by running `locale -a` on a linux system.
|
|
*
|
|
* @var array<string, string>
|
|
*/
|
|
protected array $localeMap = [
|
|
'ar' => 'ar',
|
|
'bg' => 'bg_BG',
|
|
'bs' => 'bs_BA',
|
|
'ca' => 'ca',
|
|
'cs' => 'cs_CZ',
|
|
'cy' => 'cy_GB',
|
|
'da' => 'da_DK',
|
|
'de' => 'de_DE',
|
|
'de_informal' => 'de_DE',
|
|
'el' => 'el_GR',
|
|
'en' => 'en_GB',
|
|
'es' => 'es_ES',
|
|
'es_AR' => 'es_AR',
|
|
'et' => 'et_EE',
|
|
'eu' => 'eu_ES',
|
|
'fa' => 'fa_IR',
|
|
'fr' => 'fr_FR',
|
|
'he' => 'he_IL',
|
|
'hr' => 'hr_HR',
|
|
'hu' => 'hu_HU',
|
|
'id' => 'id_ID',
|
|
'it' => 'it_IT',
|
|
'ja' => 'ja',
|
|
'ka' => 'ka_GE',
|
|
'ko' => 'ko_KR',
|
|
'lt' => 'lt_LT',
|
|
'lv' => 'lv_LV',
|
|
'nb' => 'nb_NO',
|
|
'nl' => 'nl_NL',
|
|
'pl' => 'pl_PL',
|
|
'pt' => 'pt_PT',
|
|
'pt_BR' => 'pt_BR',
|
|
'ro' => 'ro_RO',
|
|
'ru' => 'ru',
|
|
'sk' => 'sk_SK',
|
|
'sl' => 'sl_SI',
|
|
'sv' => 'sv_SE',
|
|
'tr' => 'tr_TR',
|
|
'uk' => 'uk_UA',
|
|
'uz' => 'uz_UZ',
|
|
'vi' => 'vi_VN',
|
|
'zh_CN' => 'zh_CN',
|
|
'zh_TW' => 'zh_TW',
|
|
];
|
|
|
|
/**
|
|
* Get the BookStack locale string for the given user.
|
|
*/
|
|
protected function getLocaleForUser(User $user): string
|
|
{
|
|
$default = config('app.default_locale');
|
|
|
|
if ($user->isGuest() && config('app.auto_detect_locale')) {
|
|
return $this->autoDetectLocale(request(), $default);
|
|
}
|
|
|
|
return setting()->getUser($user, 'language', $default);
|
|
}
|
|
|
|
/**
|
|
* Get a locale definition for the current user.
|
|
*/
|
|
public function getForUser(User $user): LocaleDefinition
|
|
{
|
|
$localeString = $this->getLocaleForUser($user);
|
|
|
|
return new LocaleDefinition(
|
|
$localeString,
|
|
$this->localeMap[$localeString] ?? $localeString,
|
|
in_array($localeString, $this->rtlLocales),
|
|
);
|
|
}
|
|
|
|
/**
|
|
* Autodetect the visitors locale by matching locales in their headers
|
|
* against the locales supported by BookStack.
|
|
*/
|
|
protected function autoDetectLocale(Request $request, string $default): string
|
|
{
|
|
$availableLocales = $this->getAllAppLocales();
|
|
|
|
foreach ($request->getLanguages() as $lang) {
|
|
if (in_array($lang, $availableLocales)) {
|
|
return $lang;
|
|
}
|
|
}
|
|
|
|
return $default;
|
|
}
|
|
|
|
/**
|
|
* Get all the available app-specific level locale strings.
|
|
*/
|
|
public function getAllAppLocales(): array
|
|
{
|
|
return array_keys($this->localeMap);
|
|
}
|
|
}
|