mirror of
https://github.com/BookStackApp/BookStack.git
synced 2024-10-01 01:36:00 -04:00
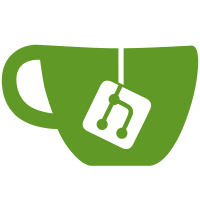
- Added mulit-level depth parsing. - Updating usage of HTML doc in page content to be efficient. - Removed now redundant PageContentTest cases. - Made some include system fixes based upon testing.
69 lines
1.4 KiB
PHP
69 lines
1.4 KiB
PHP
<?php
|
|
|
|
namespace BookStack\Entities\Tools;
|
|
|
|
use BookStack\Util\HtmlDocument;
|
|
use DOMNode;
|
|
|
|
class PageIncludeContent
|
|
{
|
|
protected static array $topLevelTags = ['table', 'ul', 'ol', 'pre'];
|
|
|
|
/**
|
|
* @var DOMNode[]
|
|
*/
|
|
protected array $contents = [];
|
|
|
|
protected bool $isTopLevel = false;
|
|
|
|
public function __construct(
|
|
string $html,
|
|
PageIncludeTag $tag,
|
|
) {
|
|
$this->parseHtml($html, $tag);
|
|
}
|
|
|
|
protected function parseHtml(string $html, PageIncludeTag $tag): void
|
|
{
|
|
if (empty($html)) {
|
|
return;
|
|
}
|
|
|
|
$doc = new HtmlDocument($html);
|
|
|
|
$sectionId = $tag->getSectionId();
|
|
if (!$sectionId) {
|
|
$this->contents = [...$doc->getBodyChildren()];
|
|
$this->isTopLevel = true;
|
|
return;
|
|
}
|
|
|
|
$section = $doc->getElementById($sectionId);
|
|
if (!$section) {
|
|
return;
|
|
}
|
|
|
|
$isTopLevel = in_array(strtolower($section->nodeName), static::$topLevelTags);
|
|
$this->isTopLevel = $isTopLevel;
|
|
$this->contents = $isTopLevel ? [$section] : [...$section->childNodes];
|
|
}
|
|
|
|
public function isInline(): bool
|
|
{
|
|
return !$this->isTopLevel;
|
|
}
|
|
|
|
public function isEmpty(): bool
|
|
{
|
|
return empty($this->contents);
|
|
}
|
|
|
|
/**
|
|
* @return DOMNode[]
|
|
*/
|
|
public function toDomNodes(): array
|
|
{
|
|
return $this->contents;
|
|
}
|
|
}
|