mirror of
https://github.com/BookStackApp/BookStack.git
synced 2024-09-13 04:52:38 +00:00
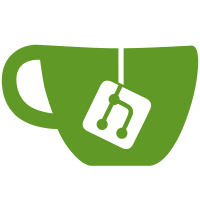
- Intended to prevent enumeration to check if a user exists. - Updated messages on both the reqest-reset and set-password elements. - Also updated notification auto-hide to be dynamic based upon the amount of words within the notification. - Added tests to cover. For #2016
74 lines
2.1 KiB
PHP
74 lines
2.1 KiB
PHP
<?php
|
|
|
|
namespace BookStack\Http\Controllers\Auth;
|
|
|
|
use BookStack\Http\Controllers\Controller;
|
|
use Illuminate\Foundation\Auth\ResetsPasswords;
|
|
use Illuminate\Http\Request;
|
|
use Illuminate\Support\Facades\Password;
|
|
|
|
class ResetPasswordController extends Controller
|
|
{
|
|
/*
|
|
|--------------------------------------------------------------------------
|
|
| Password Reset Controller
|
|
|--------------------------------------------------------------------------
|
|
|
|
|
| This controller is responsible for handling password reset requests
|
|
| and uses a simple trait to include this behavior. You're free to
|
|
| explore this trait and override any methods you wish to tweak.
|
|
|
|
|
*/
|
|
|
|
use ResetsPasswords;
|
|
|
|
protected $redirectTo = '/';
|
|
|
|
/**
|
|
* Create a new controller instance.
|
|
*
|
|
* @return void
|
|
*/
|
|
public function __construct()
|
|
{
|
|
$this->middleware('guest');
|
|
$this->middleware('guard:standard');
|
|
parent::__construct();
|
|
}
|
|
|
|
/**
|
|
* Get the response for a successful password reset.
|
|
*
|
|
* @param Request $request
|
|
* @param string $response
|
|
* @return \Illuminate\Http\Response
|
|
*/
|
|
protected function sendResetResponse(Request $request, $response)
|
|
{
|
|
$message = trans('auth.reset_password_success');
|
|
$this->showSuccessNotification($message);
|
|
return redirect($this->redirectPath())
|
|
->with('status', trans($response));
|
|
}
|
|
|
|
/**
|
|
* Get the response for a failed password reset.
|
|
*
|
|
* @param \Illuminate\Http\Request $request
|
|
* @param string $response
|
|
* @return \Illuminate\Http\RedirectResponse|\Illuminate\Http\JsonResponse
|
|
*/
|
|
protected function sendResetFailedResponse(Request $request, $response)
|
|
{
|
|
// We show invalid users as invalid tokens as to not leak what
|
|
// users may exist in the system.
|
|
if ($response === Password::INVALID_USER) {
|
|
$response = Password::INVALID_TOKEN;
|
|
}
|
|
|
|
return redirect()->back()
|
|
->withInput($request->only('email'))
|
|
->withErrors(['email' => trans($response)]);
|
|
}
|
|
}
|