mirror of
https://github.com/BookStackApp/BookStack.git
synced 2024-08-25 03:35:35 +00:00
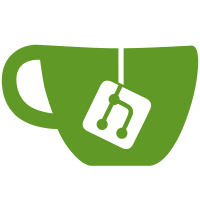
- Intended to prevent enumeration to check if a user exists. - Updated messages on both the reqest-reset and set-password elements. - Also updated notification auto-hide to be dynamic based upon the amount of words within the notification. - Added tests to cover. For #2016
50 lines
1.4 KiB
JavaScript
50 lines
1.4 KiB
JavaScript
|
|
class Notification {
|
|
|
|
constructor(elem) {
|
|
this.elem = elem;
|
|
this.type = elem.getAttribute('notification');
|
|
this.textElem = elem.querySelector('span');
|
|
this.autohide = this.elem.hasAttribute('data-autohide');
|
|
this.elem.style.display = 'grid';
|
|
|
|
window.$events.listen(this.type, text => {
|
|
this.show(text);
|
|
});
|
|
elem.addEventListener('click', this.hide.bind(this));
|
|
|
|
if (elem.hasAttribute('data-show')) {
|
|
setTimeout(() => this.show(this.textElem.textContent), 100);
|
|
}
|
|
|
|
this.hideCleanup = this.hideCleanup.bind(this);
|
|
}
|
|
|
|
show(textToShow = '') {
|
|
this.elem.removeEventListener('transitionend', this.hideCleanup);
|
|
this.textElem.textContent = textToShow;
|
|
this.elem.style.display = 'grid';
|
|
setTimeout(() => {
|
|
this.elem.classList.add('showing');
|
|
}, 1);
|
|
|
|
if (this.autohide) {
|
|
const words = textToShow.split(' ').length;
|
|
const timeToShow = Math.max(2000, 1000 + (250 * words));
|
|
setTimeout(this.hide.bind(this), timeToShow);
|
|
}
|
|
}
|
|
|
|
hide() {
|
|
this.elem.classList.remove('showing');
|
|
this.elem.addEventListener('transitionend', this.hideCleanup);
|
|
}
|
|
|
|
hideCleanup() {
|
|
this.elem.style.display = 'none';
|
|
this.elem.removeEventListener('transitionend', this.hideCleanup);
|
|
}
|
|
|
|
}
|
|
|
|
export default Notification; |