mirror of
https://github.com/BookStackApp/BookStack.git
synced 2024-10-01 01:36:00 -04:00
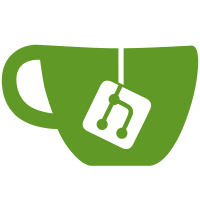
Decided it's relevant to entity updated_at since tags are now indexed alongside content. - Also fixed tags not applied on shelf. - Also enforced proper page API update validation. - Adds tests to cover. For #3319 Fixes #3370
92 lines
2.3 KiB
PHP
92 lines
2.3 KiB
PHP
<?php
|
|
|
|
namespace BookStack\Entities\Repos;
|
|
|
|
use BookStack\Actions\TagRepo;
|
|
use BookStack\Entities\Models\Entity;
|
|
use BookStack\Entities\Models\HasCoverImage;
|
|
use BookStack\Exceptions\ImageUploadException;
|
|
use BookStack\Uploads\ImageRepo;
|
|
use Illuminate\Http\UploadedFile;
|
|
|
|
class BaseRepo
|
|
{
|
|
protected TagRepo $tagRepo;
|
|
protected ImageRepo $imageRepo;
|
|
|
|
public function __construct(TagRepo $tagRepo, ImageRepo $imageRepo)
|
|
{
|
|
$this->tagRepo = $tagRepo;
|
|
$this->imageRepo = $imageRepo;
|
|
}
|
|
|
|
/**
|
|
* Create a new entity in the system.
|
|
*/
|
|
public function create(Entity $entity, array $input)
|
|
{
|
|
$entity->fill($input);
|
|
$entity->forceFill([
|
|
'created_by' => user()->id,
|
|
'updated_by' => user()->id,
|
|
'owned_by' => user()->id,
|
|
]);
|
|
$entity->refreshSlug();
|
|
$entity->save();
|
|
|
|
if (isset($input['tags'])) {
|
|
$this->tagRepo->saveTagsToEntity($entity, $input['tags']);
|
|
}
|
|
|
|
$entity->rebuildPermissions();
|
|
$entity->indexForSearch();
|
|
}
|
|
|
|
/**
|
|
* Update the given entity.
|
|
*/
|
|
public function update(Entity $entity, array $input)
|
|
{
|
|
$entity->fill($input);
|
|
$entity->updated_by = user()->id;
|
|
|
|
if ($entity->isDirty('name')) {
|
|
$entity->refreshSlug();
|
|
}
|
|
|
|
$entity->save();
|
|
|
|
if (isset($input['tags'])) {
|
|
$this->tagRepo->saveTagsToEntity($entity, $input['tags']);
|
|
$entity->touch();
|
|
}
|
|
|
|
$entity->rebuildPermissions();
|
|
$entity->indexForSearch();
|
|
}
|
|
|
|
/**
|
|
* Update the given items' cover image, or clear it.
|
|
*
|
|
* @param Entity&HasCoverImage $entity
|
|
*
|
|
* @throws ImageUploadException
|
|
* @throws \Exception
|
|
*/
|
|
public function updateCoverImage($entity, ?UploadedFile $coverImage, bool $removeImage = false)
|
|
{
|
|
if ($coverImage) {
|
|
$this->imageRepo->destroyImage($entity->cover);
|
|
$image = $this->imageRepo->saveNew($coverImage, 'cover_book', $entity->id, 512, 512, true);
|
|
$entity->cover()->associate($image);
|
|
$entity->save();
|
|
}
|
|
|
|
if ($removeImage) {
|
|
$this->imageRepo->destroyImage($entity->cover);
|
|
$entity->image_id = 0;
|
|
$entity->save();
|
|
}
|
|
}
|
|
}
|