mirror of
https://github.com/BookStackApp/BookStack.git
synced 2024-07-16 16:22:44 +00:00
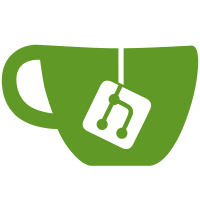
Changed to be rendered server side along with page content. Changed deletion to fully delete comments from the database. Added 'local_id' to comments for referencing. Updated reply system to be non-nested (Incomplete) Made database comment format entity-agnostic to be more future proof. Updated designs of comment sections.
44 lines
971 B
PHP
44 lines
971 B
PHP
<?php namespace BookStack;
|
|
|
|
class Comment extends Ownable
|
|
{
|
|
protected $fillable = ['text', 'html', 'parent_id'];
|
|
protected $appends = ['created', 'updated'];
|
|
|
|
/**
|
|
* Get the entity that this comment belongs to
|
|
* @return \Illuminate\Database\Eloquent\Relations\MorphTo
|
|
*/
|
|
public function entity()
|
|
{
|
|
return $this->morphTo('entity');
|
|
}
|
|
|
|
/**
|
|
* Check if a comment has been updated since creation.
|
|
* @return bool
|
|
*/
|
|
public function isUpdated()
|
|
{
|
|
return $this->updated_at->timestamp > $this->created_at->timestamp;
|
|
}
|
|
|
|
/**
|
|
* Get created date as a relative diff.
|
|
* @return mixed
|
|
*/
|
|
public function getCreatedAttribute()
|
|
{
|
|
return $this->created_at->diffForHumans();
|
|
}
|
|
|
|
/**
|
|
* Get updated date as a relative diff.
|
|
* @return mixed
|
|
*/
|
|
public function getUpdatedAttribute()
|
|
{
|
|
return $this->updated_at->diffForHumans();
|
|
}
|
|
}
|