mirror of
https://github.com/BookStackApp/BookStack.git
synced 2024-08-24 03:05:37 +00:00
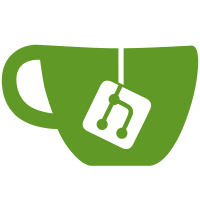
* Started mass-refactoring of the current entity repos * Rewrote book tree logic - Now does two simple queries instead of one really complex one. - Extracted logic into its own class. - Remove model-level akward union field listing. - Logic now more readable than being large separate query and compilation functions. * Extracted and split book sort logic * Finished up Book controller/repo organisation * Refactored bookshelves controllers and repo parts * Fixed issues found via phpunit * Refactored Chapter controller * Updated Chapter export controller * Started Page controller/repo refactor * Refactored another chunk of PageController * Completed initial pagecontroller refactor pass * Fixed tests and continued reduction of old repos * Removed old page remove and further reduced entity repo * Removed old entity repo, split out page controller * Ran phpcbf and split out some page content methods * Tidied up some EntityProvider elements * Fixed issued caused by viewservice change
63 lines
1.6 KiB
PHP
63 lines
1.6 KiB
PHP
<?php namespace BookStack\Entities;
|
|
|
|
class SlugGenerator
|
|
{
|
|
|
|
protected $entity;
|
|
|
|
/**
|
|
* SlugGenerator constructor.
|
|
* @param $entity
|
|
*/
|
|
public function __construct(Entity $entity)
|
|
{
|
|
$this->entity = $entity;
|
|
}
|
|
|
|
/**
|
|
* Generate a fresh slug for the given entity.
|
|
* The slug will generated so it does not conflict within the same parent item.
|
|
*/
|
|
public function generate(): string
|
|
{
|
|
$slug = $this->formatNameAsSlug($this->entity->name);
|
|
while ($this->slugInUse($slug)) {
|
|
$slug .= '-' . substr(md5(rand(1, 500)), 0, 3);
|
|
}
|
|
return $slug;
|
|
}
|
|
|
|
/**
|
|
* Format a name as a url slug.
|
|
*/
|
|
protected function formatNameAsSlug(string $name): string
|
|
{
|
|
$slug = preg_replace('/[\+\/\\\?\@\}\{\.\,\=\[\]\#\&\!\*\'\;\:\$\%]/', '', mb_strtolower($name));
|
|
$slug = preg_replace('/\s{2,}/', ' ', $slug);
|
|
$slug = str_replace(' ', '-', $slug);
|
|
if ($slug === "") {
|
|
$slug = substr(md5(rand(1, 500)), 0, 5);
|
|
}
|
|
return $slug;
|
|
}
|
|
|
|
/**
|
|
* Check if a slug is already in-use for this
|
|
* type of model within the same parent.
|
|
*/
|
|
protected function slugInUse(string $slug): bool
|
|
{
|
|
$query = $this->entity->newQuery()->where('slug', '=', $slug);
|
|
|
|
if ($this->entity instanceof BookChild) {
|
|
$query->where('book_id', '=', $this->entity->book_id);
|
|
}
|
|
|
|
if ($this->entity->id) {
|
|
$query->where('id', '!=', $this->entity->id);
|
|
}
|
|
|
|
return $query->count() > 0;
|
|
}
|
|
}
|