mirror of
https://github.com/BookStackApp/BookStack.git
synced 2024-08-24 11:15:56 +00:00
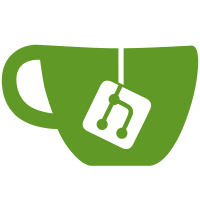
* Started mass-refactoring of the current entity repos * Rewrote book tree logic - Now does two simple queries instead of one really complex one. - Extracted logic into its own class. - Remove model-level akward union field listing. - Logic now more readable than being large separate query and compilation functions. * Extracted and split book sort logic * Finished up Book controller/repo organisation * Refactored bookshelves controllers and repo parts * Fixed issues found via phpunit * Refactored Chapter controller * Updated Chapter export controller * Started Page controller/repo refactor * Refactored another chunk of PageController * Completed initial pagecontroller refactor pass * Fixed tests and continued reduction of old repos * Removed old page remove and further reduced entity repo * Removed old entity repo, split out page controller * Ran phpcbf and split out some page content methods * Tidied up some EntityProvider elements * Fixed issued caused by viewservice change
61 lines
1.5 KiB
PHP
61 lines
1.5 KiB
PHP
<?php namespace BookStack\Entities;
|
|
|
|
use Illuminate\Database\Eloquent\Builder;
|
|
use Illuminate\Database\Eloquent\Relations\BelongsTo;
|
|
|
|
/**
|
|
* Class BookChild
|
|
* @property int $book_id
|
|
* @property int $priority
|
|
* @property Book $book
|
|
* @method Builder whereSlugs(string $bookSlug, string $childSlug)
|
|
*/
|
|
class BookChild extends Entity
|
|
{
|
|
|
|
/**
|
|
* Scope a query to find items where the the child has the given childSlug
|
|
* where its parent has the bookSlug.
|
|
*/
|
|
public function scopeWhereSlugs(Builder $query, string $bookSlug, string $childSlug)
|
|
{
|
|
return $query->with('book')
|
|
->whereHas('book', function (Builder $query) use ($bookSlug) {
|
|
$query->where('slug', '=', $bookSlug);
|
|
})
|
|
->where('slug', '=', $childSlug);
|
|
}
|
|
|
|
/**
|
|
* Get the book this page sits in.
|
|
* @return BelongsTo
|
|
*/
|
|
public function book(): BelongsTo
|
|
{
|
|
return $this->belongsTo(Book::class);
|
|
}
|
|
|
|
/**
|
|
* Change the book that this entity belongs to.
|
|
*/
|
|
public function changeBook(int $newBookId): Entity
|
|
{
|
|
$this->book_id = $newBookId;
|
|
$this->refreshSlug();
|
|
$this->save();
|
|
$this->refresh();
|
|
|
|
// Update related activity
|
|
$this->activity()->update(['book_id' => $newBookId]);
|
|
|
|
// Update all child pages if a chapter
|
|
if ($this instanceof Chapter) {
|
|
foreach ($this->pages as $page) {
|
|
$page->changeBook($newBookId);
|
|
}
|
|
}
|
|
|
|
return $this;
|
|
}
|
|
}
|