mirror of
https://github.com/BookStackApp/BookStack.git
synced 2024-08-26 20:22:18 +00:00
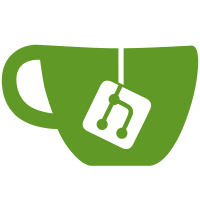
The runtime userCan() and the JointPermissionBuilder now share much of the same logic for handling entity permission resolution.
136 lines
4.3 KiB
PHP
136 lines
4.3 KiB
PHP
<?php
|
|
|
|
namespace BookStack\Auth\Permissions;
|
|
|
|
use BookStack\Auth\Role;
|
|
use BookStack\Entities\Models\Entity;
|
|
use Illuminate\Database\Eloquent\Builder;
|
|
|
|
class EntityPermissionEvaluator
|
|
{
|
|
protected string $action;
|
|
|
|
public function __construct(string $action)
|
|
{
|
|
$this->action = $action;
|
|
}
|
|
|
|
public function evaluateEntityForUser(Entity $entity, array $userRoleIds): ?bool
|
|
{
|
|
if ($this->isUserSystemAdmin($userRoleIds)) {
|
|
return true;
|
|
}
|
|
|
|
$typeIdChain = $this->gatherEntityChainTypeIds(SimpleEntityData::fromEntity($entity));
|
|
$relevantPermissions = $this->getPermissionsMapByTypeId($typeIdChain, [...$userRoleIds, 0]);
|
|
$permitsByType = $this->collapseAndCategorisePermissions($typeIdChain, $relevantPermissions);
|
|
|
|
return $this->evaluatePermitsByType($permitsByType);
|
|
}
|
|
|
|
/**
|
|
* @param array<string, array<string, int>> $permitsByType
|
|
*/
|
|
protected function evaluatePermitsByType(array $permitsByType): ?bool
|
|
{
|
|
// Return grant or reject from role-level if exists
|
|
if (count($permitsByType['role']) > 0) {
|
|
return boolval(max($permitsByType['role']));
|
|
}
|
|
|
|
// Return fallback permission if exists
|
|
if (count($permitsByType['fallback']) > 0) {
|
|
return boolval($permitsByType['fallback'][0]);
|
|
}
|
|
|
|
return null;
|
|
}
|
|
|
|
/**
|
|
* @param string[] $typeIdChain
|
|
* @param array<string, EntityPermission[]> $permissionMapByTypeId
|
|
* @return array<string, array<string, int>>
|
|
*/
|
|
protected function collapseAndCategorisePermissions(array $typeIdChain, array $permissionMapByTypeId): array
|
|
{
|
|
$permitsByType = ['fallback' => [], 'role' => []];
|
|
|
|
foreach ($typeIdChain as $typeId) {
|
|
$permissions = $permissionMapByTypeId[$typeId] ?? [];
|
|
foreach ($permissions as $permission) {
|
|
$roleId = $permission->role_id;
|
|
$type = $roleId === 0 ? 'fallback' : 'role';
|
|
if (!isset($permitsByType[$type][$roleId])) {
|
|
$permitsByType[$type][$roleId] = $permission->{$this->action};
|
|
}
|
|
}
|
|
}
|
|
|
|
return $permitsByType;
|
|
}
|
|
|
|
/**
|
|
* @param string[] $typeIdChain
|
|
* @return array<string, EntityPermission[]>
|
|
*/
|
|
protected function getPermissionsMapByTypeId(array $typeIdChain, array $filterRoleIds): array
|
|
{
|
|
$query = EntityPermission::query()->where(function (Builder $query) use ($typeIdChain) {
|
|
foreach ($typeIdChain as $typeId) {
|
|
$query->orWhere(function (Builder $query) use ($typeId) {
|
|
[$type, $id] = explode(':', $typeId);
|
|
$query->where('entity_type', '=', $type)
|
|
->where('entity_id', '=', $id);
|
|
});
|
|
}
|
|
});
|
|
|
|
if (!empty($filterRoleIds)) {
|
|
$query->where(function (Builder $query) use ($filterRoleIds) {
|
|
$query->whereIn('role_id', [...$filterRoleIds, 0]);
|
|
});
|
|
}
|
|
|
|
$relevantPermissions = $query->get(['entity_id', 'entity_type', 'role_id', $this->action])->all();
|
|
|
|
$map = [];
|
|
foreach ($relevantPermissions as $permission) {
|
|
$key = $permission->entity_type . ':' . $permission->entity_id;
|
|
if (!isset($map[$key])) {
|
|
$map[$key] = [];
|
|
}
|
|
|
|
$map[$key][] = $permission;
|
|
}
|
|
|
|
return $map;
|
|
}
|
|
|
|
/**
|
|
* @return string[]
|
|
*/
|
|
protected function gatherEntityChainTypeIds(SimpleEntityData $entity): array
|
|
{
|
|
// The array order here is very important due to the fact we walk up the chain
|
|
// elsewhere in the class. Earlier items in the chain have higher priority.
|
|
|
|
$chain = [$entity->type . ':' . $entity->id];
|
|
|
|
if ($entity->type === 'page' && $entity->chapter_id) {
|
|
$chain[] = 'chapter:' . $entity->chapter_id;
|
|
}
|
|
|
|
if ($entity->type === 'page' || $entity->type === 'chapter') {
|
|
$chain[] = 'book:' . $entity->book_id;
|
|
}
|
|
|
|
return $chain;
|
|
}
|
|
|
|
protected function isUserSystemAdmin($userRoleIds): bool
|
|
{
|
|
$adminRoleId = Role::getSystemRole('admin')->id;
|
|
return in_array($adminRoleId, $userRoleIds);
|
|
}
|
|
}
|