mirror of
https://github.com/BookStackApp/BookStack.git
synced 2024-10-01 01:36:00 -04:00
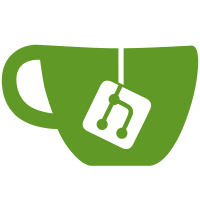
- Added fadeIn to animation JS service. - Updated overlay to use anim service and to recieve a callback for after-anim actions. - Updated code editor popup to refresh Codemirror instance layout after animation has completed. Fixes #1672
45 lines
1.1 KiB
JavaScript
45 lines
1.1 KiB
JavaScript
import codeLib from "../services/code";
|
|
|
|
const methods = {
|
|
show() {
|
|
if (!this.editor) this.editor = codeLib.popupEditor(this.$refs.editor, this.language);
|
|
this.$refs.overlay.components.overlay.show(() => {
|
|
codeLib.updateLayout(this.editor);
|
|
});
|
|
},
|
|
hide() {
|
|
this.$refs.overlay.components.overlay.hide();
|
|
},
|
|
updateEditorMode(language) {
|
|
codeLib.setMode(this.editor, language, this.editor.getValue());
|
|
},
|
|
updateLanguage(lang) {
|
|
this.language = lang;
|
|
this.updateEditorMode(lang);
|
|
},
|
|
open(code, language, callback) {
|
|
this.show();
|
|
this.updateEditorMode(language);
|
|
this.language = language;
|
|
codeLib.setContent(this.editor, code);
|
|
this.code = code;
|
|
this.callback = callback;
|
|
},
|
|
save() {
|
|
if (!this.callback) return;
|
|
this.callback(this.editor.getValue(), this.language);
|
|
this.hide();
|
|
}
|
|
};
|
|
|
|
const data = {
|
|
editor: null,
|
|
language: '',
|
|
code: '',
|
|
callback: null
|
|
};
|
|
|
|
export default {
|
|
methods,
|
|
data
|
|
}; |