mirror of
https://github.com/BookStackApp/BookStack.git
synced 2024-10-01 01:36:00 -04:00
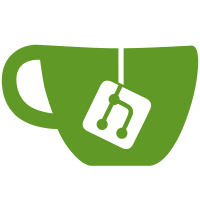
Created modified TestResponse so we can use DOM operations in new Testcases as we move away from the BrowserKit tests.
63 lines
1.6 KiB
PHP
63 lines
1.6 KiB
PHP
<?php namespace Tests;
|
|
|
|
use Illuminate\Foundation\Testing\DatabaseTransactions;
|
|
use Illuminate\Foundation\Testing\TestCase as BaseTestCase;
|
|
|
|
abstract class TestCase extends BaseTestCase
|
|
{
|
|
use CreatesApplication;
|
|
use DatabaseTransactions;
|
|
use SharedTestHelpers;
|
|
|
|
/**
|
|
* The base URL to use while testing the application.
|
|
* @var string
|
|
*/
|
|
protected $baseUrl = 'http://localhost';
|
|
|
|
/**
|
|
* Assert a permission error has occurred.
|
|
* @param TestResponse $response
|
|
* @return TestCase
|
|
*/
|
|
protected function assertPermissionError(TestResponse $response)
|
|
{
|
|
$response->assertRedirect('/');
|
|
$this->assertSessionHas('error');
|
|
session()->remove('error');
|
|
return $this;
|
|
}
|
|
|
|
/**
|
|
* Assert the session contains a specific entry.
|
|
* @param string $key
|
|
* @return $this
|
|
*/
|
|
protected function assertSessionHas(string $key)
|
|
{
|
|
$this->assertTrue(session()->has($key), "Session does not contain a [{$key}] entry");
|
|
return $this;
|
|
}
|
|
|
|
/**
|
|
* Override of the get method so we can get visibility of custom TestResponse methods.
|
|
* @param string $uri
|
|
* @param array $headers
|
|
* @return TestResponse
|
|
*/
|
|
public function get($uri, array $headers = [])
|
|
{
|
|
return parent::get($uri, $headers);
|
|
}
|
|
|
|
/**
|
|
* Create the test response instance from the given response.
|
|
*
|
|
* @param \Illuminate\Http\Response $response
|
|
* @return TestResponse
|
|
*/
|
|
protected function createTestResponse($response)
|
|
{
|
|
return TestResponse::fromBaseResponse($response);
|
|
}
|
|
} |