mirror of
https://repo.getmonero.org/AnonDev/xmrmemes.git
synced 2025-04-26 10:19:15 -04:00
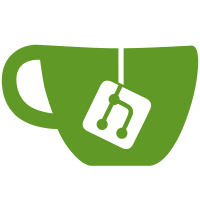
Add dark / light theme Add social sharing on meme pages Add approving / pending admin section Improve design Add pagination on profiles Make front end date time user friendly Finish rough draft of site And Much more... Still need to fix a few minor things before it goes live. Almost complete.
101 lines
3.6 KiB
PHP
101 lines
3.6 KiB
PHP
<?php
|
|
|
|
namespace App\Jobs;
|
|
|
|
use Illuminate\Bus\Queueable;
|
|
use Illuminate\Contracts\Queue\ShouldBeUnique;
|
|
use Illuminate\Contracts\Queue\ShouldQueue;
|
|
use Illuminate\Foundation\Bus\Dispatchable;
|
|
use Illuminate\Queue\InteractsWithQueue;
|
|
use Illuminate\Queue\SerializesModels;
|
|
|
|
use App\Models\Tip;
|
|
use App\Models\Address;
|
|
use App\Models\Meme;
|
|
use MoneroIntegrations\MoneroPhp\walletRPC;
|
|
|
|
// Can put code below inside functions to debug the Monero library
|
|
// ini_set('display_errors', 1);
|
|
// ini_set('display_startup_errors', 1);
|
|
// error_reporting(E_ALL);
|
|
|
|
class ProcessPayments implements ShouldQueue
|
|
{
|
|
use Dispatchable, InteractsWithQueue, Queueable, SerializesModels;
|
|
|
|
/**
|
|
* Create a new job instance.
|
|
*
|
|
* @return void
|
|
*/
|
|
public function __construct()
|
|
{
|
|
//
|
|
}
|
|
|
|
/**
|
|
* Execute the job.
|
|
*
|
|
* @return void
|
|
*/
|
|
public function handle()
|
|
{
|
|
$this->get_transactions();
|
|
$this->payout();
|
|
}
|
|
|
|
public function get_transactions()
|
|
{
|
|
try {
|
|
$walletRPC = new walletRPC('127.0.0.1', config('app.xmr_network_port')); // Change to match your wallet (monero-wallet-rpc) IP address and port; 18083 is the customary port for mainnet, 28083 for testnet, 38083 for stagenet
|
|
$open_wallet = $walletRPC->open_wallet(config('app.xmr_wallet_name'), '');
|
|
$get_transfers = $walletRPC->get_transfers('all', true);
|
|
if (isset($get_transfers['in'])) {
|
|
foreach ($get_transfers['in'] as $transfer) {
|
|
$address = Address::where('address', $transfer['address'])->first();
|
|
$tip_exists = Tip::where('txid', $transfer['txid'])->first();
|
|
if ($address && !$tip_exists) {
|
|
$tip = new Tip();
|
|
$tip->address_id = $address->id;
|
|
$tip->amount = $transfer['amount'];
|
|
$tip->txid = $transfer['txid'];
|
|
$tip->is_deposit = 1;
|
|
$tip->save();
|
|
$meme = Meme::where('address_id', $address->id)->firstOrFail();
|
|
$meme->payment_pending = 1;
|
|
$meme->save();
|
|
}
|
|
}
|
|
}
|
|
$walletRPC->close_wallet();
|
|
} catch (\Exception $e) {
|
|
echo 'Caught exception: ', $e->getMessage(), "\n";
|
|
}
|
|
}
|
|
|
|
public function payout()
|
|
{
|
|
try {
|
|
$walletRPC = new walletRPC('127.0.0.1', config('app.xmr_network_port')); // Change to match your wallet (monero-wallet-rpc) IP address and port; 18083 is the customary port for mainnet, 28083 for testnet, 38083 for stagenet
|
|
$open_wallet = $walletRPC->open_wallet(config('app.xmr_wallet_name'), '');
|
|
$meme = Meme::where('payment_pending', 1)->firstOrFail();
|
|
if ($meme->user->address) {
|
|
$send_funds = $walletRPC->sweep_all($meme->user->address, $meme->address->address_index);
|
|
if ($send_funds['amount_list']) {
|
|
$tip = new Tip;
|
|
$tip->address_id = $meme->address_id;
|
|
$tip->amount = $send_funds['amount_list'][0];
|
|
$tip->txid = $send_funds['tx_hash_list'][0];
|
|
$tip->is_deposit = 0;
|
|
$tip->save();
|
|
$meme->payment_pending = 0;
|
|
$meme->save();
|
|
}
|
|
}
|
|
$walletRPC->close_wallet();
|
|
} catch (\Exception $e) {
|
|
echo 'Caught exception: ', $e->getMessage(), "\n";
|
|
}
|
|
}
|
|
}
|