mirror of
https://repo.getmonero.org/AnonDev/xmrmemes.git
synced 2025-05-20 18:00:21 -04:00
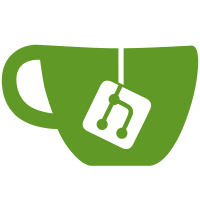
The sweep_all seems to throw timeout errors sometimes (not always) which causes saving the outbound transaction to fail which is very problematic. Now we will run that last and save the outbound transactions before hand. This hopefully should fix the issue
115 lines
4.5 KiB
PHP
115 lines
4.5 KiB
PHP
<?php
|
|
|
|
namespace App\Jobs;
|
|
|
|
use Illuminate\Bus\Queueable;
|
|
use Illuminate\Contracts\Queue\ShouldBeUnique;
|
|
use Illuminate\Contracts\Queue\ShouldQueue;
|
|
use Illuminate\Foundation\Bus\Dispatchable;
|
|
use Illuminate\Queue\InteractsWithQueue;
|
|
use Illuminate\Queue\SerializesModels;
|
|
|
|
use App\Models\Tip;
|
|
use App\Models\Address;
|
|
use App\Models\Meme;
|
|
use MoneroIntegrations\MoneroPhp\walletRPC;
|
|
|
|
class ProcessPayments implements ShouldQueue
|
|
{
|
|
use Dispatchable, InteractsWithQueue, Queueable, SerializesModels;
|
|
|
|
public $timeout = 3600;
|
|
|
|
/**
|
|
* Create a new job instance.
|
|
*
|
|
* @return void
|
|
*/
|
|
public function __construct()
|
|
{
|
|
//
|
|
}
|
|
|
|
/**
|
|
* Execute the job.
|
|
*
|
|
* @return void
|
|
*/
|
|
public function handle()
|
|
{
|
|
$this->get_transactions();
|
|
$this->payout();
|
|
}
|
|
|
|
public function get_transactions()
|
|
{
|
|
try {
|
|
$walletRPC = new walletRPC(config('app.xmr_daemon_ip'), config('app.xmr_network_port')); // Change to match your wallet (monero-wallet-rpc) IP address and port; 18083 is the customary port for mainnet, 28083 for testnet, 38083 for stagenet
|
|
$open_wallet = $walletRPC->open_wallet(config('app.xmr_wallet_name'), '');
|
|
$account_indexes = Meme::pluck('account_index')->toArray();
|
|
foreach ($account_indexes as $account_index) {
|
|
// Outbound tips
|
|
$get_transfers_out = $walletRPC->get_transfers('out', $account_index);
|
|
if (isset($get_transfers_out['out'])) {
|
|
foreach ($get_transfers_out['out'] as $transfer) {
|
|
$meme_out = Meme::where('address', $transfer['address'])->first();
|
|
$tip_exists_out = Tip::where('txid', $transfer['txid'])->first();
|
|
if ($meme_out && !$tip_exists_out) {
|
|
$tip_out = new Tip();
|
|
$tip_out->meme_id = $meme_out->id;
|
|
$tip_out->amount = $transfer['amount'];
|
|
$tip_out->txid = $transfer['txid'];
|
|
$tip_out->is_deposit = 0;
|
|
$tip_out->save();
|
|
$meme_out->payment_pending = 0;
|
|
$meme_out->save();
|
|
}
|
|
}
|
|
}
|
|
// Inbound tips
|
|
$get_transfers = $walletRPC->get_transfers('in', $account_index);
|
|
if (isset($get_transfers['in'])) {
|
|
foreach ($get_transfers['in'] as $transfer) {
|
|
$meme = Meme::where('address', $transfer['address'])->first();
|
|
$tip_exists = Tip::where('txid', $transfer['txid'])->first();
|
|
if ($meme && !$tip_exists) {
|
|
$tip = new Tip();
|
|
$tip->meme_id = $meme->id;
|
|
$tip->amount = $transfer['amount'];
|
|
$tip->txid = $transfer['txid'];
|
|
$tip->is_deposit = 1;
|
|
$tip->save();
|
|
$meme->payment_pending = 1;
|
|
$meme->save();
|
|
}
|
|
}
|
|
}
|
|
}
|
|
$walletRPC->close_wallet();
|
|
} catch (\Exception $e) {
|
|
echo 'Caught exception: ', $e->getMessage(), "\n";
|
|
}
|
|
}
|
|
|
|
public function payout()
|
|
{
|
|
try {
|
|
$walletRPC = new walletRPC(config('app.xmr_daemon_ip'), config('app.xmr_network_port')); // Change to match your wallet (monero-wallet-rpc) IP address and port; 18083 is the customary port for mainnet, 28083 for testnet, 38083 for stagenet
|
|
$open_wallet = $walletRPC->open_wallet(config('app.xmr_wallet_name'), '');
|
|
$memes = Meme::where('payment_pending', 1)->get();
|
|
foreach ($memes as $meme) {
|
|
$balance = $walletRPC->get_balance($meme->account_index);
|
|
if ($balance['unlocked_balance'] > 0 && $balance['balance'] === $balance['unlocked_balance']) {
|
|
try {
|
|
$send_funds = $walletRPC->sweep_all($meme->user->address, '', $meme->account_index);
|
|
} catch (\Exception $e) {
|
|
echo 'Caught exception: ', $e->getMessage(), "\n";
|
|
}
|
|
}
|
|
}
|
|
$walletRPC->close_wallet();
|
|
} catch (\Exception $e) {
|
|
echo 'Caught exception: ', $e->getMessage(), "\n";
|
|
}
|
|
}
|
|
}
|