mirror of
https://repo.getmonero.org/AnonDev/xmrmemes.git
synced 2025-01-04 20:30:55 -05:00
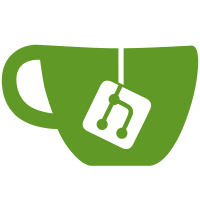
Very small amounts were showing scientific notation numbers when dispaying the total amount of tips on a meme or user or on the leaderboard. This should fix that. There may be a cleaner way to do this but this gets the job done.
79 lines
1.7 KiB
PHP
79 lines
1.7 KiB
PHP
<?php
|
|
|
|
namespace App\Models;
|
|
|
|
use Illuminate\Contracts\Auth\MustVerifyEmail;
|
|
use Illuminate\Database\Eloquent\Factories\HasFactory;
|
|
use Illuminate\Foundation\Auth\User as Authenticatable;
|
|
use Illuminate\Notifications\Notifiable;
|
|
|
|
class User extends Authenticatable
|
|
{
|
|
use HasFactory, Notifiable;
|
|
|
|
protected $appends = ['tips_count', 'tips_total', 'memes_count'];
|
|
|
|
/**
|
|
* The attributes that are mass assignable.
|
|
*
|
|
* @var array
|
|
*/
|
|
protected $fillable = [
|
|
'name',
|
|
'email',
|
|
'address',
|
|
'password',
|
|
];
|
|
|
|
/**
|
|
* The attributes that should be hidden for arrays.
|
|
*
|
|
* @var array
|
|
*/
|
|
protected $hidden = [
|
|
'password',
|
|
'remember_token',
|
|
];
|
|
|
|
/**
|
|
* The attributes that should be cast to native types.
|
|
*
|
|
* @var array
|
|
*/
|
|
protected $casts = [
|
|
'email_verified_at' => 'datetime',
|
|
];
|
|
|
|
public function memes()
|
|
{
|
|
return $this->hasMany(Meme::class)->orderByDesc('created_at');
|
|
}
|
|
|
|
public function tips()
|
|
{
|
|
return $this->hasManyThrough(Tip::class, Meme::class, 'user_id');
|
|
}
|
|
|
|
public function getMemesCountAttribute()
|
|
{
|
|
return $this->memes->count();
|
|
}
|
|
|
|
public function getTipsCountAttribute()
|
|
{
|
|
return $this->tips->where('is_deposit', 1)->count();
|
|
}
|
|
|
|
public function getTipsTotalAttribute()
|
|
{
|
|
if ($this->tips->where('is_deposit', 1)->sum('amount_formatted')) {
|
|
return rtrim(sprintf('%.8f',floatval(
|
|
$this->tips->where('is_deposit', 1)->sum('amount_formatted')
|
|
)),'0');
|
|
} else {
|
|
return 0;
|
|
}
|
|
}
|
|
|
|
}
|