mirror of
https://github.com/comit-network/xmr-btc-swap.git
synced 2025-07-02 02:26:57 -04:00
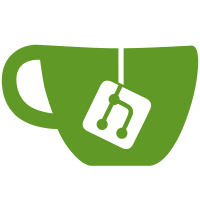
On Linux and macOS, no program output was being observed. This is referenced in the [LocalTime] docs for `tracing-subscriber`, which links to this [unsoundness issue] in the time crate. Rather than introducing a possible vector for undefined behaviour and segfaults, I have just changed the logging to use UTC time instead. When running the ASB as a systemd service, one would generally use the `--disable-timestamps` flag anyway as systemd adds its own timestamps which can be local to the server. If the situation with `tracing-subscriber` and the time crate is fixed then this can be updated. This commit also updates the `tracing-subscriber` and `tracing-appender` dependencies, closing #987. [LocalTime]: https://docs.rs/tracing-subscriber/latest/tracing_subscriber/fmt/time/struct.LocalTime.html [unsoundness issue]: https://github.com/time-rs/time/issues/293#issuecomment-748151025
65 lines
1.7 KiB
Rust
65 lines
1.7 KiB
Rust
#![allow(clippy::unwrap_used)] // This is only meant to be used in tests.
|
|
|
|
use std::io;
|
|
use std::sync::{Arc, Mutex};
|
|
use tracing::subscriber;
|
|
use tracing_subscriber::filter::LevelFilter;
|
|
use tracing_subscriber::fmt::MakeWriter;
|
|
|
|
/// Setup tracing with a capturing writer, allowing assertions on the log
|
|
/// messages.
|
|
///
|
|
/// Time and ANSI are disabled to make the output more predictable and
|
|
/// readable.
|
|
pub fn capture_logs(min_level: LevelFilter) -> MakeCapturingWriter {
|
|
let make_writer = MakeCapturingWriter::default();
|
|
|
|
let guard = subscriber::set_default(
|
|
tracing_subscriber::fmt()
|
|
.with_ansi(false)
|
|
.without_time()
|
|
.with_writer(make_writer.clone())
|
|
.with_env_filter(format!("{}", min_level))
|
|
.finish(),
|
|
);
|
|
// don't clean up guard we stay initialized
|
|
std::mem::forget(guard);
|
|
|
|
make_writer
|
|
}
|
|
|
|
#[derive(Default, Clone)]
|
|
pub struct MakeCapturingWriter {
|
|
writer: CapturingWriter,
|
|
}
|
|
|
|
impl MakeCapturingWriter {
|
|
pub fn captured(&self) -> String {
|
|
let captured = &self.writer.captured;
|
|
let cursor = captured.lock().unwrap();
|
|
String::from_utf8(cursor.clone().into_inner()).unwrap()
|
|
}
|
|
}
|
|
|
|
impl<'a> MakeWriter<'a> for MakeCapturingWriter {
|
|
type Writer = CapturingWriter;
|
|
|
|
fn make_writer(&self) -> Self::Writer {
|
|
self.writer.clone()
|
|
}
|
|
}
|
|
|
|
#[derive(Default, Clone)]
|
|
pub struct CapturingWriter {
|
|
captured: Arc<Mutex<io::Cursor<Vec<u8>>>>,
|
|
}
|
|
|
|
impl io::Write for CapturingWriter {
|
|
fn write(&mut self, buf: &[u8]) -> io::Result<usize> {
|
|
self.captured.lock().unwrap().write(buf)
|
|
}
|
|
|
|
fn flush(&mut self) -> io::Result<()> {
|
|
Ok(())
|
|
}
|
|
}
|