mirror of
https://github.com/comit-network/xmr-btc-swap.git
synced 2024-07-01 00:51:23 +00:00
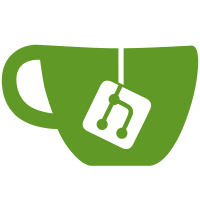
Since Alice's refund scenario starts with generating the temporary wallet from keys to claim the XMR which results in Alice' unloading the wallet. Alice then loads her original wallet to be able to handle more swaps. Since Alice is in the role of the long running daemon handling concurrent swaps, the operation to close, claim and re-open her default wallet must be atomic. This PR adds an additional step, that sweeps all the refunded XMR back into the default wallet. In order to ensure that this is possible, Alice has to ensure that the locked XMR got enough confirmations. These changes allow us to assert Alice's balance after refunding.
66 lines
2.0 KiB
Rust
66 lines
2.0 KiB
Rust
pub mod testutils;
|
|
|
|
use swap::protocol::bob::BobState;
|
|
use swap::protocol::{alice, bob};
|
|
use testutils::bob_run_until::is_btc_locked;
|
|
use testutils::FastCancelConfig;
|
|
|
|
#[tokio::test]
|
|
async fn given_bob_manually_refunds_after_btc_locked_bob_refunds() {
|
|
testutils::setup_test(FastCancelConfig, |mut ctx| async move {
|
|
let (bob_swap, bob_join_handle) = ctx.bob_swap().await;
|
|
let bob_swap = tokio::spawn(bob::run_until(bob_swap, is_btc_locked));
|
|
|
|
let alice_swap = ctx.alice_next_swap().await;
|
|
let alice_swap = tokio::spawn(alice::run(alice_swap));
|
|
|
|
let bob_state = bob_swap.await??;
|
|
assert!(matches!(bob_state, BobState::BtcLocked { .. }));
|
|
|
|
let (bob_swap, bob_join_handle) = ctx.stop_and_resume_bob_from_db(bob_join_handle).await;
|
|
|
|
// Ensure Bob's timelock is expired
|
|
if let BobState::BtcLocked(state3) = bob_swap.state.clone() {
|
|
state3
|
|
.wait_for_cancel_timelock_to_expire(bob_swap.bitcoin_wallet.as_ref())
|
|
.await?;
|
|
} else {
|
|
panic!("Bob in unexpected state {}", bob_swap.state);
|
|
}
|
|
|
|
// Bob manually cancels
|
|
bob_join_handle.abort();
|
|
let (_, state) = bob::cancel(
|
|
bob_swap.swap_id,
|
|
bob_swap.state,
|
|
bob_swap.bitcoin_wallet,
|
|
bob_swap.db,
|
|
false,
|
|
)
|
|
.await??;
|
|
assert!(matches!(state, BobState::BtcCancelled { .. }));
|
|
|
|
let (bob_swap, bob_join_handle) = ctx.stop_and_resume_bob_from_db(bob_join_handle).await;
|
|
assert!(matches!(bob_swap.state, BobState::BtcCancelled { .. }));
|
|
|
|
// Bob manually refunds
|
|
bob_join_handle.abort();
|
|
let bob_state = bob::refund(
|
|
bob_swap.swap_id,
|
|
bob_swap.state,
|
|
bob_swap.bitcoin_wallet,
|
|
bob_swap.db,
|
|
false,
|
|
)
|
|
.await??;
|
|
|
|
ctx.assert_bob_refunded(bob_state).await;
|
|
|
|
let alice_state = alice_swap.await??;
|
|
ctx.assert_alice_refunded(alice_state).await;
|
|
|
|
Ok(())
|
|
})
|
|
.await
|
|
}
|