mirror of
https://github.com/comit-network/xmr-btc-swap.git
synced 2024-10-01 05:45:40 +00:00
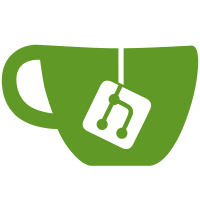
It might very well be that the cancel transaction is already published. If that is the case, there is no point in failing the command. We simply transition to cancel and exit normally. The reason this comes up now is because Alice now properly waits for the cancel timelock as well and publishes the cancel transaction first. Ultimately, she should not do that because there is no benefit to her unless she can also publish the punish transaction.
65 lines
2.1 KiB
Rust
65 lines
2.1 KiB
Rust
use crate::bitcoin::{ExpiredTimelocks, Txid, Wallet};
|
|
use crate::database::{Database, Swap};
|
|
use crate::protocol::bob::BobState;
|
|
use anyhow::{bail, Result};
|
|
use std::sync::Arc;
|
|
use uuid::Uuid;
|
|
|
|
#[derive(Debug, thiserror::Error, Clone, Copy)]
|
|
pub enum Error {
|
|
#[error("The cancel timelock has not expired yet.")]
|
|
CancelTimelockNotExpiredYet,
|
|
}
|
|
|
|
pub async fn cancel(
|
|
swap_id: Uuid,
|
|
state: BobState,
|
|
bitcoin_wallet: Arc<Wallet>,
|
|
db: Database,
|
|
force: bool,
|
|
) -> Result<Result<(Txid, BobState), Error>> {
|
|
let state6 = match state {
|
|
BobState::BtcLocked(state3) => state3.cancel(),
|
|
BobState::XmrLockProofReceived { state, .. } => state.cancel(),
|
|
BobState::XmrLocked(state4) => state4.cancel(),
|
|
BobState::EncSigSent(state4) => state4.cancel(),
|
|
BobState::CancelTimelockExpired(state6) => state6,
|
|
BobState::Started { .. }
|
|
| BobState::ExecutionSetupDone(_)
|
|
| BobState::BtcRedeemed(_)
|
|
| BobState::BtcCancelled(_)
|
|
| BobState::BtcRefunded(_)
|
|
| BobState::XmrRedeemed { .. }
|
|
| BobState::BtcPunished { .. }
|
|
| BobState::SafelyAborted => bail!(
|
|
"Cannot cancel swap {} because it is in state {} which is not refundable.",
|
|
swap_id,
|
|
state
|
|
),
|
|
};
|
|
|
|
tracing::info!(%swap_id, "Manually cancelling swap");
|
|
|
|
if !force {
|
|
tracing::debug!(%swap_id, "Checking if cancel timelock is expired");
|
|
|
|
if let ExpiredTimelocks::None = state6.expired_timelock(bitcoin_wallet.as_ref()).await? {
|
|
return Ok(Err(Error::CancelTimelockNotExpiredYet));
|
|
}
|
|
}
|
|
|
|
let txid = if let Ok(tx) = state6.check_for_tx_cancel(bitcoin_wallet.as_ref()).await {
|
|
tracing::debug!(%swap_id, "Cancel transaction has already been published");
|
|
|
|
tx.txid()
|
|
} else {
|
|
state6.submit_tx_cancel(bitcoin_wallet.as_ref()).await?
|
|
};
|
|
|
|
let state = BobState::BtcCancelled(state6);
|
|
let db_state = state.clone().into();
|
|
db.insert_latest_state(swap_id, Swap::Bob(db_state)).await?;
|
|
|
|
Ok(Ok((txid, state)))
|
|
}
|