mirror of
https://github.com/comit-network/xmr-btc-swap.git
synced 2024-10-01 01:45:40 -04:00
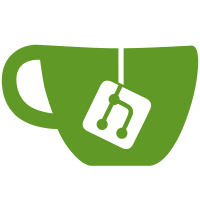
- Properly check the timelocks before trying to redeem - Distinguish different failure scenarios and reactions to it. - if we fail to construct the redeem transaction: wait for cancel. - if we fail to publish the redeem transaction: wait for cancel but let the user know that restarting the application will result in retrying to publish the tx. - if we succeed to publish the tx but then fail when waiting for finality, print error to the user (secreat already leaked, the user has to check manually if the tx was included)
36 lines
1.3 KiB
Rust
36 lines
1.3 KiB
Rust
use swap::protocol::{alice, alice::AliceState, bob};
|
|
|
|
pub mod testutils;
|
|
|
|
/// Bob locks btc and Alice locks xmr. Alice fails to act so Bob refunds. Alice
|
|
/// is forced to refund even though she learned the secret and would be able to
|
|
/// redeem had the timelock not expired.
|
|
#[tokio::test]
|
|
async fn given_alice_restarts_after_enc_sig_learned_and_bob_already_cancelled_refund_swap() {
|
|
testutils::setup_test(|mut ctx| async move {
|
|
let alice_swap = ctx.new_swap_as_alice().await;
|
|
let bob_swap = ctx.new_swap_as_bob().await;
|
|
|
|
let bob = bob::run(bob_swap);
|
|
let bob_handle = tokio::spawn(bob);
|
|
|
|
let alice_state = alice::run_until(alice_swap, alice::swap::is_encsig_learned)
|
|
.await
|
|
.unwrap();
|
|
assert!(matches!(alice_state, AliceState::EncSigLearned {..}));
|
|
|
|
// Wait for Bob to refund, because Alice does not act
|
|
let bob_state = bob_handle.await.unwrap();
|
|
ctx.assert_bob_refunded(bob_state.unwrap()).await;
|
|
|
|
// Once bob has finished Alice is restarted and refunds as well
|
|
let alice_swap = ctx.recover_alice_from_db().await;
|
|
assert!(matches!(alice_swap.state, AliceState::EncSigLearned {..}));
|
|
|
|
let alice_state = alice::run(alice_swap).await.unwrap();
|
|
|
|
ctx.assert_alice_refunded(alice_state).await;
|
|
})
|
|
.await;
|
|
}
|