mirror of
https://github.com/comit-network/xmr-btc-swap.git
synced 2025-07-01 18:17:04 -04:00
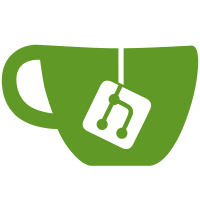
We now, - emit a Tauri event when the Monero lock transaction receives a new confirmation - emit a Tauri event with a list of transaction hashes once we have published the Monero redeem transaction - gui: display the confirmations and txids This PR closes #12.
47 lines
1.2 KiB
TypeScript
47 lines
1.2 KiB
TypeScript
import { Link, Typography } from "@material-ui/core";
|
|
import { ReactNode } from "react";
|
|
import InfoBox from "./InfoBox";
|
|
|
|
export type TransactionInfoBoxProps = {
|
|
title: string;
|
|
txId: string | null;
|
|
explorerUrlCreator: ((txId: string) => string) | null;
|
|
additionalContent: ReactNode;
|
|
loading: boolean;
|
|
icon: JSX.Element;
|
|
};
|
|
|
|
export default function TransactionInfoBox({
|
|
title,
|
|
txId,
|
|
additionalContent,
|
|
icon,
|
|
loading,
|
|
explorerUrlCreator,
|
|
}: TransactionInfoBoxProps) {
|
|
return (
|
|
<InfoBox
|
|
title={title}
|
|
mainContent={
|
|
<Typography variant="h5">
|
|
{txId ?? "Transaction ID not available"}
|
|
</Typography>
|
|
}
|
|
loading={loading}
|
|
additionalContent={
|
|
<>
|
|
<Typography variant="subtitle2">{additionalContent}</Typography>
|
|
{explorerUrlCreator != null &&
|
|
txId != null && ( // Only show the link if the txId is not null and we have a creator for the explorer URL
|
|
<Typography variant="body1">
|
|
<Link href={explorerUrlCreator(txId)} target="_blank">
|
|
View on explorer
|
|
</Link>
|
|
</Typography>
|
|
)}
|
|
</>
|
|
}
|
|
icon={icon}
|
|
/>
|
|
);
|
|
}
|