mirror of
https://github.com/comit-network/xmr-btc-swap.git
synced 2024-07-02 17:41:33 +00:00
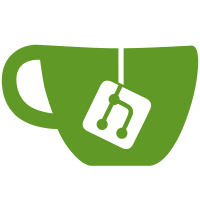
As Bob is dialing Alice, we now ensure that we are connected to Alice at each step that needs communication. If we are not connected, we proceed with dialing. In an attempt to improve libp2p usage, we also add known address of Alice first and only use peer_id to dial. This ensures that we use the expected peer id.
124 lines
3.4 KiB
Rust
124 lines
3.4 KiB
Rust
use crate::testutils::{init_alice, init_bob};
|
|
use futures::future::try_join;
|
|
use get_port::get_port;
|
|
use libp2p::Multiaddr;
|
|
use rand::rngs::OsRng;
|
|
use swap::{alice, bob};
|
|
use testcontainers::clients::Cli;
|
|
use testutils::init_tracing;
|
|
use uuid::Uuid;
|
|
use xmr_btc::{bitcoin, config::Config};
|
|
|
|
pub mod testutils;
|
|
|
|
/// Run the following tests with RUST_MIN_STACK=10000000
|
|
|
|
#[tokio::test]
|
|
async fn happy_path() {
|
|
let _guard = init_tracing();
|
|
|
|
let cli = Cli::default();
|
|
let (
|
|
monero,
|
|
testutils::Containers {
|
|
bitcoind,
|
|
monerods: _monerods,
|
|
},
|
|
) = testutils::init_containers(&cli).await;
|
|
|
|
let btc_to_swap = bitcoin::Amount::from_sat(1_000_000);
|
|
let btc_alice = bitcoin::Amount::ZERO;
|
|
let btc_bob = btc_to_swap * 10;
|
|
|
|
// this xmr value matches the logic of alice::calculate_amounts i.e. btc *
|
|
// 10_000 * 100
|
|
let xmr_to_swap = xmr_btc::monero::Amount::from_piconero(1_000_000_000_000);
|
|
let xmr_alice = xmr_to_swap * 10;
|
|
let xmr_bob = xmr_btc::monero::Amount::ZERO;
|
|
|
|
let port = get_port().expect("Failed to find a free port");
|
|
let alice_multiaddr: Multiaddr = format!("/ip4/127.0.0.1/tcp/{}", port)
|
|
.parse()
|
|
.expect("failed to parse Alice's address");
|
|
|
|
let config = Config::regtest();
|
|
|
|
let (
|
|
alice_state,
|
|
mut alice_event_loop,
|
|
alice_event_loop_handle,
|
|
alice_btc_wallet,
|
|
alice_xmr_wallet,
|
|
alice_db,
|
|
) = init_alice(
|
|
&bitcoind,
|
|
&monero,
|
|
btc_to_swap,
|
|
xmr_to_swap,
|
|
xmr_alice,
|
|
alice_multiaddr.clone(),
|
|
config,
|
|
)
|
|
.await;
|
|
|
|
let (bob_state, bob_event_loop, bob_event_loop_handle, bob_btc_wallet, bob_xmr_wallet, bob_db) =
|
|
init_bob(
|
|
alice_multiaddr.clone(),
|
|
alice_event_loop.peer_id(),
|
|
&bitcoind,
|
|
&monero,
|
|
btc_to_swap,
|
|
btc_bob,
|
|
xmr_to_swap,
|
|
config,
|
|
)
|
|
.await;
|
|
|
|
let alice_swap_fut = alice::swap::swap(
|
|
alice_state,
|
|
alice_event_loop_handle,
|
|
alice_btc_wallet.clone(),
|
|
alice_xmr_wallet.clone(),
|
|
config,
|
|
Uuid::new_v4(),
|
|
alice_db,
|
|
);
|
|
|
|
let alice_peer_id = alice_event_loop.peer_id();
|
|
|
|
let _alice_swarm_fut = tokio::spawn(async move { alice_event_loop.run().await });
|
|
|
|
let bob_swap_fut = bob::swap::swap(
|
|
bob_state,
|
|
bob_event_loop_handle,
|
|
bob_db,
|
|
bob_btc_wallet.clone(),
|
|
bob_xmr_wallet.clone(),
|
|
OsRng,
|
|
Uuid::new_v4(),
|
|
alice_peer_id,
|
|
alice_multiaddr,
|
|
);
|
|
|
|
let _bob_swarm_fut = tokio::spawn(async move { bob_event_loop.run().await });
|
|
|
|
try_join(alice_swap_fut, bob_swap_fut).await.unwrap();
|
|
|
|
let btc_alice_final = alice_btc_wallet.as_ref().balance().await.unwrap();
|
|
let btc_bob_final = bob_btc_wallet.as_ref().balance().await.unwrap();
|
|
|
|
let xmr_alice_final = alice_xmr_wallet.as_ref().get_balance().await.unwrap();
|
|
|
|
bob_xmr_wallet.as_ref().0.refresh().await.unwrap();
|
|
let xmr_bob_final = bob_xmr_wallet.as_ref().get_balance().await.unwrap();
|
|
|
|
assert_eq!(
|
|
btc_alice_final,
|
|
btc_alice + btc_to_swap - bitcoin::Amount::from_sat(bitcoin::TX_FEE)
|
|
);
|
|
assert!(btc_bob_final <= btc_bob - btc_to_swap);
|
|
|
|
assert!(xmr_alice_final <= xmr_alice - xmr_to_swap);
|
|
assert_eq!(xmr_bob_final, xmr_bob + xmr_to_swap);
|
|
}
|