mirror of
https://github.com/tillitis/tillitis-key1.git
synced 2025-07-18 12:48:45 -04:00
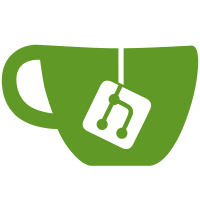
This is an import of the fw-2 tag of tkey-libs. We import the entire tkey-libs repo minus dot files into the tillitis-key1 repo to make it very simple not to make mistakes regarding which firmware tag depends on which tkey-libs tag, especially considering locking down with NVCM. Please see README for information about developing with another tkey-libs or how to import future tkey-libs. Since tkey-libs is now a part of the repo we also add tkey-libs to the clean_fw target.
51 lines
861 B
C
51 lines
861 B
C
// SPDX-FileCopyrightText: 2022 Tillitis AB <tillitis.se>
|
|
// SPDX-License-Identifier: BSD-2-Clause
|
|
|
|
#include <stddef.h>
|
|
#include <stdint.h>
|
|
#include <tkey/assert.h>
|
|
#include <tkey/debug.h>
|
|
#include <tkey/proto.h>
|
|
#include <tkey/tk1_mem.h>
|
|
|
|
uint8_t genhdr(uint8_t id, uint8_t endpoint, uint8_t status, enum cmdlen len)
|
|
{
|
|
return (id << 5) | (endpoint << 3) | (status << 2) | len;
|
|
}
|
|
|
|
int parseframe(uint8_t b, struct frame_header *hdr)
|
|
{
|
|
if ((b & 0x80) != 0) {
|
|
// Bad version
|
|
return -1;
|
|
}
|
|
|
|
if ((b & 0x4) != 0) {
|
|
// Must be 0
|
|
return -1;
|
|
}
|
|
|
|
hdr->id = (b & 0x60) >> 5;
|
|
hdr->endpoint = (b & 0x18) >> 3;
|
|
|
|
// Length
|
|
switch (b & 0x3) {
|
|
case LEN_1:
|
|
hdr->len = 1;
|
|
break;
|
|
case LEN_4:
|
|
hdr->len = 4;
|
|
break;
|
|
case LEN_32:
|
|
hdr->len = 32;
|
|
break;
|
|
case LEN_128:
|
|
hdr->len = 128;
|
|
break;
|
|
default:
|
|
// Unknown length
|
|
return -1;
|
|
}
|
|
|
|
return 0;
|
|
}
|