mirror of
https://github.com/ben-grande/qusal.git
synced 2024-12-15 02:44:31 -05:00
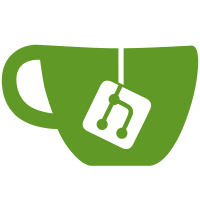
Echo can interpret operand as an option and checking every variable to be echoed is troublesome while with printf, if the format specifier is present before the operand, printing as string can be enforced.
351 lines
10 KiB
Bash
Executable File
351 lines
10 KiB
Bash
Executable File
#!/bin/sh
|
|
|
|
# SPDX-FileCopyrightText: 2015 - 2020 Marek Marczykowski-Gorecki <marmarek@invisiblethingslab.com>
|
|
# SPDX-FileCopyrightText: 2024 Benjamin Grande M. S. <ben.grande.b@gmail.com>
|
|
#
|
|
# SPDX-License-Identifier: GPL-2.0-only
|
|
|
|
## Description: rewrite repositories definitions to be used with the cacher.
|
|
## It works for qubes that should be configured to use the cacher via Qrexec
|
|
## or Netvm (direct networking).
|
|
##
|
|
## Looping through files and testing their permissions (read, write) is better
|
|
## than finding a file and trying to sed it without knowledge, it is also
|
|
## beneficial as 'find' fails if file is not existent and sending all 'find'
|
|
## output to /dev/stderr is not great.
|
|
##
|
|
## Assigning the repositories files to '${@}' avoids having to parse their
|
|
## names in case they contain spaces, newlines and other dangerous characters
|
|
## to the shell, it is also an easy way to use an array for /bin/sh.
|
|
|
|
set -eu
|
|
|
|
set_proxy_marker(){
|
|
marker_begin_text="QUBES BEGIN"
|
|
marker_end_text="QUBES END"
|
|
marker_begin="### ${marker_begin_text} ###"
|
|
marker_end="### ${marker_end_text} ###"
|
|
proxy_file="${1}"
|
|
proxy_options="${2}"
|
|
|
|
if ! grep -q -e "^${marker_begin}$" -- "${proxy_file}"; then
|
|
if grep -q -e "^${marker_end}$" -- "${proxy_file}"; then
|
|
msg="found marker ${marker_end_text} but not ${marker_begin_text}"
|
|
msg="${msg} in ${proxy_file}."
|
|
msg="${msg} fix it by removing markers or adding missing ones and retry"
|
|
printf '%s\n' "Error: ${msg}" >&2
|
|
exit 1
|
|
fi
|
|
cp -- "${proxy_file}" "${proxy_file}.qubes-orig"
|
|
printf '%s\n' "${marker_begin}" | tee -a -- "${proxy_file}" >/dev/null
|
|
printf '%s\n' "${marker_end}" | tee -a -- "${proxy_file}" >/dev/null
|
|
elif ! grep -q -e "^${marker_end}$" -- "${proxy_file}"; then
|
|
msg="found marker ${marker_begin_text} but not ${marker_end_text}"
|
|
msg="${msg} in ${proxy_file}."
|
|
msg="${msg} fix it by removing markers or adding missing ones and retry"
|
|
printf '%s\n' "error: ${msg}" >&2
|
|
exit 1
|
|
fi
|
|
|
|
proxy_tmp_file="$(mktemp)"
|
|
cat >"${proxy_tmp_file}" <<EOF
|
|
# The text between ${marker_begin_text} and ${marker_end_text} is
|
|
# automatically generated by $0. All changes here will be overridden.
|
|
# You can override options after the ${marker_end_text}.
|
|
${proxy_options}
|
|
EOF
|
|
|
|
## Couldn't figure out how to write only changes on the next sed.
|
|
if ! grep -q -e "${proxy_options}" -- "${proxy_file}"; then
|
|
tee -a -- "${changes_file}" <"${proxy_tmp_file}" >/dev/null
|
|
fi
|
|
## GNU Sed, only reliable while we don't support BSD.
|
|
sed -i -e "/^${marker_begin}$/,/^${marker_end}$/{
|
|
/^${marker_end}$/b
|
|
/^${marker_begin}$/!d
|
|
r ${proxy_tmp_file}
|
|
}" -- "${proxy_file}"
|
|
rm -f -- "${proxy_tmp_file}"
|
|
}
|
|
|
|
check_netvm_cacher(){
|
|
proxy_host="127.0.0.1"
|
|
proxy_port="8082"
|
|
proxy_addr=""
|
|
proxy_url=""
|
|
proxy_conf=""
|
|
if ! test -f /var/run/qubes-service/updates-proxy-setup; then
|
|
return 0
|
|
fi
|
|
if test -f /var/run/qubes-service/netvm-cacher; then
|
|
proxy_host="$(qubesdb-read /qubes-gateway)"
|
|
if test -z "${proxy_host}"; then
|
|
printf '%s\n' \
|
|
"Error: service netvm-cacher enabled but netvm IP not found" >&2
|
|
return 1
|
|
fi
|
|
fi
|
|
proxy_addr="${proxy_host}:${proxy_port}"
|
|
proxy_url="http://${proxy_addr}"
|
|
proxy_conf="proxy=${proxy_addr}"
|
|
}
|
|
|
|
reject_os(){
|
|
msg_unsupported="${0##*/} does not support your OS distribution."
|
|
printf '%s\n' "${msg_unsupported}" >&2
|
|
exit 1
|
|
}
|
|
|
|
# shellcheck disable=SC2317
|
|
set_proxy_os(){
|
|
if test -e /etc/fedora-release; then
|
|
## Fedora
|
|
|
|
if test -w /etc/dnf/dnf.conf; then
|
|
set_proxy_marker /etc/dnf/dnf.conf "zchunk=False
|
|
${proxy_conf}"
|
|
fi
|
|
if test -n "${proxy_addr}"; then
|
|
cat >/etc/yum.conf.d/qubes-proxy.conf <<EOF
|
|
${proxy_conf}
|
|
EOF
|
|
else
|
|
rm -f -- /etc/yum.conf.d/qubes-proxy.conf
|
|
fi
|
|
|
|
set --
|
|
for repo in \
|
|
/etc/yum.repos.d/*.repo
|
|
do
|
|
test -f "${repo}" || continue
|
|
test -r "${repo}" || continue
|
|
test -w "${repo}" || continue
|
|
set -- "${@}" "${repo}"
|
|
done
|
|
test -n "${*}" || return 0
|
|
|
|
case "${action}" in
|
|
install)
|
|
baseurl_search="baseurl\s*=\s*https://"
|
|
baseurl_repl="baseurl=http://HTTPS///"
|
|
meta_search="metalink\s*=\s*https://"
|
|
meta_repl="metalink=http://HTTPS///"
|
|
baseurl_expr="s|${baseurl_search}|${baseurl_repl}|w ${changes_file}"
|
|
meta_expr="s|${meta_search}|${meta_repl}|w ${changes_file}"
|
|
find "${@}" -type f -exec sed -i \
|
|
-e "${baseurl_expr}" -e "${meta_expr}" \
|
|
-- {} \+
|
|
|
|
set --
|
|
for repo in \
|
|
/etc/yum.repos.d/rpmfusion*.repo
|
|
do
|
|
test -f "${repo}" || continue
|
|
test -r "${repo}" || continue
|
|
test -w "${repo}" || continue
|
|
set -- "${@}" "${repo}"
|
|
done
|
|
test -n "${*}" || return 0
|
|
|
|
baseurl_search="^\s*#.*baseurl"
|
|
baseurl_repl="baseurl"
|
|
meta_search="^\s*metalink\s*=\s*"
|
|
meta_expr="#metalink="
|
|
baseurl_expr="s|${baseurl_search}|${baseurl_repl}|w ${changes_file}"
|
|
meta_expr="s|${meta_search}|${meta_repl}|w ${changes_file}"
|
|
find "${@}" -type f -exec sed -i \
|
|
-e "${baseurl_expr}" -e "${meta_expr}" \
|
|
-- {} \+
|
|
;;
|
|
|
|
uninstall)
|
|
baseurl_search="baseurl\s*=\s*http://HTTPS///"
|
|
baseurl_repl="baseurl=https://"
|
|
meta_search="metalink\s*=\s*http://HTTPS///"
|
|
meta_repl="metalink=https://"
|
|
baseurl_expr="s|${baseurl_search}|${baseurl_repl}|w ${changes_file}"
|
|
meta_expr="s|${meta_search}|${meta_repl}|w ${changes_file}"
|
|
find "${@}" -type f -exec sed -i \
|
|
-e "${baseurl_expr}" -e "${meta_expr}" \
|
|
-- {} \+
|
|
|
|
set --
|
|
for repo in \
|
|
/etc/yum.repos.d/rpmfusion*.repo
|
|
do
|
|
test -f "${repo}" || continue
|
|
test -r "${repo}" || continue
|
|
test -w "${repo}" || continue
|
|
set -- "${@}" "${repo}"
|
|
done
|
|
test -n "${*}" || return 0
|
|
|
|
find "${@}" -type f -exec sed -i \
|
|
-e "s|^\s*baseurl|#baseurl|w ${changes_file}" \
|
|
-e "s|^\s*#.*metalink\s*=|metalink=|w ${changes_file}" \
|
|
-- {} \+ 2>/dev/null || true
|
|
;;
|
|
*) printf '%s\n' "Unsupported action" >&2; exit 1
|
|
esac
|
|
|
|
elif test -e /etc/debian_version && test ! -e /usr/share/whonix/marker; then
|
|
## Debian and derivatives but not Whonix.
|
|
|
|
if test -n "${proxy_addr}"; then
|
|
cat >/etc/apt/apt.conf.d/50cacher-proxy <<EOF
|
|
# Use Cacher NetVM Update Proxy
|
|
Acquire::http::Proxy "${proxy_url}";
|
|
Acquire::tor::proxy "${proxy_url}";
|
|
EOF
|
|
else
|
|
rm -f -- /etc/apt/apt.conf.d/50cacher-proxy
|
|
fi
|
|
|
|
set --
|
|
for repo in \
|
|
/etc/apt/sources.list \
|
|
/etc/apt/sources.list.d/*.list \
|
|
/etc/apt/sources.list.d/*.sources
|
|
do
|
|
test -f "${repo}" || continue
|
|
test -r "${repo}" || continue
|
|
test -w "${repo}" || continue
|
|
set -- "${@}" "${repo}"
|
|
done
|
|
test -n "${*}" || return 0
|
|
|
|
case "${action}" in
|
|
install)
|
|
sources_search="URIs:\s*https://"
|
|
sources_repl="URIs: http://HTTPS///"
|
|
list_search="^\s*\(#*\)\s*deb\(.*\)https://"
|
|
list_repl="\1deb\2http://HTTPS///"
|
|
sources_expr="s|${sources_search}|${sources_repl}|w ${changes_file}"
|
|
list_expr="s|${list_search}|${list_repl}|w ${changes_file}"
|
|
find "${@}" -type f -exec sed -i \
|
|
-e "${list_expr}" -e "${sources_expr}" \
|
|
-- {} \+
|
|
;;
|
|
|
|
uninstall)
|
|
sources_search="URIs:\s*http://HTTPS///"
|
|
sources_repl="URIs: https://"
|
|
list_search="^\s*\(#*\)\s*deb\(.*\)http://HTTPS///"
|
|
list_repl="\1deb\2https://"
|
|
sources_expr="s|${sources_search}|${sources_repl}|w ${changes_file}"
|
|
list_expr="s|${list_search}|${list_repl}|w ${changes_file}"
|
|
find "${@}" -type f -exec sed -i \
|
|
-e "${list_expr}" -e "${sources_expr}" \
|
|
-- {} \+
|
|
;;
|
|
*) printf '%s\n' "Unsupported action" >&2; exit 1
|
|
esac
|
|
|
|
elif test -e /etc/arch-release; then
|
|
## Archlinux
|
|
|
|
if test -n "${proxy_addr}"; then
|
|
if ! test -d /run/qubes/bin; then
|
|
mkdir -p -- /run/qubes/bin
|
|
fi
|
|
cat >/run/qubes/bin/pacman <<EOF
|
|
#!/bin/sh
|
|
exec env ALL_PROXY="${proxy_url}" /usr/bin/pacman "\${@}"
|
|
EOF
|
|
chmod -- +x /run/qubes/bin/pacman
|
|
cat >/etc/profile.d/qubes-proxy.sh << EOF
|
|
export PATH=/run/qubes/bin:\${PATH}
|
|
EOF
|
|
else
|
|
rm -f -- /run/qubes/bin/pacman /etc/profile.d/qubes-proxy.sh
|
|
fi
|
|
|
|
set --
|
|
for repo in \
|
|
/etc/pacman.d/mirrorlist \
|
|
/etc/pacman.d/*.conf \
|
|
/etc/pacman.d/*.conf.disabled
|
|
do
|
|
test -f "${repo}" || continue
|
|
test -r "${repo}" || continue
|
|
test -w "${repo}" || continue
|
|
set -- "${@}" "${repo}"
|
|
done
|
|
test -n "${*}" || return 0
|
|
|
|
case "${action}" in
|
|
install)
|
|
repo_search="Server\s*=\s*https://" \
|
|
repo_repl="Server = http://HTTPS///"
|
|
repo_regex="s|${repo_search}|${repo_repl}|w ${changes_file}"
|
|
find "${@}" -type f -exec sed -i \
|
|
-e "${repo_regex}" \
|
|
-- {} \+
|
|
;;
|
|
|
|
uninstall)
|
|
repo_search="Server\s*=\s*http://HTTPS///"
|
|
repo_repl="Server = https://"
|
|
repo_regex="s|${repo_search}|${repo_repl}|w ${changes_file}"
|
|
find "${@}" -type f -exec sed -i \
|
|
-e "${repo_regex}" \
|
|
-- {} \+
|
|
;;
|
|
*) printf '%s\n' "Unsupported action" >&2; exit 1
|
|
esac
|
|
|
|
else
|
|
## Gentoo: upstream does not have a good solution:
|
|
## https://wiki.gentoo.org/wiki/Local_distfiles_cache#Configuring_for_Gentoo
|
|
reject_os
|
|
fi
|
|
}
|
|
|
|
set_proxy_unspecific_os(){
|
|
if test -w /etc/PackageKit/PackageKit.conf; then
|
|
set_proxy_marker /etc/PackageKit/PackageKit.conf "ProxyHTTP=${proxy_url}"
|
|
fi
|
|
}
|
|
|
|
usage(){
|
|
printf '%s\n' "Usage: ${0##*/} [install|uninstall]"
|
|
printf '%s\n' "Note: autodetection occurs if not argument is specified"
|
|
exit 1
|
|
}
|
|
|
|
changes_file="$(mktemp)"
|
|
trap 'rm -f -- "${changes_file}"' HUP INT QUIT ABRT EXIT
|
|
|
|
if test -f /var/run/qubes-service/updates-proxy-setup ||
|
|
test -f /var/run/qubes-service/netvm-cacher
|
|
then
|
|
action="install"
|
|
else
|
|
action="uninstall"
|
|
fi
|
|
|
|
case "${1-}" in
|
|
install|uninstall) action="${1}";;
|
|
"") ;;
|
|
*) usage;;
|
|
esac
|
|
|
|
uid="$(id -u)"
|
|
if test "${uid}" != "0"; then
|
|
printf '%s\n' "Error: Permission denied, action requires root privileges."
|
|
exit 1
|
|
fi
|
|
|
|
check_netvm_cacher
|
|
set_proxy_os
|
|
set_proxy_unspecific_os
|
|
|
|
## Stateful Salt cmd Module.
|
|
printf '\n'
|
|
if test -s "${changes_file}"; then
|
|
printf '%s\n' "changed=yes comment='configuration was modified'"
|
|
else
|
|
printf '%s\n' "changed=no comment='configuration remained untouched'"
|
|
fi
|
|
exit
|