mirror of
https://github.com/mirage/qubes-mirage-firewall.git
synced 2024-06-29 07:32:21 +00:00
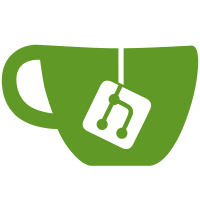
We don't need the GUI anyway. Error was: Fatal error: exception Failure("End-of-file from GUId in dom0") Raised at file "pervasives.ml", line 30, characters 22-33 Called from file "src/core/lwt.ml", line 754, characters 44-47 Mirage exiting with status 2 Do_exit called!
92 lines
3.4 KiB
OCaml
92 lines
3.4 KiB
OCaml
(* Copyright (C) 2015, Thomas Leonard <thomas.leonard@unikernel.com>
|
|
See the README file for details. *)
|
|
|
|
open Lwt
|
|
open Qubes
|
|
|
|
let src = Logs.Src.create "unikernel" ~doc:"Main unikernel code"
|
|
module Log = (val Logs.src_log src : Logs.LOG)
|
|
|
|
(* Configure logging *)
|
|
let () =
|
|
let open Logs in
|
|
(* Set default log level *)
|
|
set_level (Some Logs.Info)
|
|
|
|
module Main (Clock : V1.CLOCK) = struct
|
|
module Logs_reporter = Mirage_logs.Make(Clock)
|
|
module Uplink = Uplink.Make(Clock)
|
|
|
|
(* Set up networking and listen for incoming packets. *)
|
|
let network qubesDB =
|
|
(* Read configuration from QubesDB *)
|
|
let config = Dao.read_network_config qubesDB in
|
|
Logs.info (fun f -> f "Client (internal) network is %a"
|
|
Ipaddr.V4.Prefix.pp_hum config.Dao.clients_prefix);
|
|
(* Initialise connection to NetVM *)
|
|
Uplink.connect config >>= fun uplink ->
|
|
(* Report success *)
|
|
Dao.set_iptables_error qubesDB "" >>= fun () ->
|
|
(* Set up client-side networking *)
|
|
let client_eth = Client_eth.create
|
|
~client_gw:config.Dao.clients_our_ip
|
|
~prefix:config.Dao.clients_prefix in
|
|
(* Set up routing between networks and hosts *)
|
|
let router = Router.create
|
|
~client_eth
|
|
~uplink:(Uplink.interface uplink) in
|
|
(* Handle packets from both networks *)
|
|
Lwt.join [
|
|
Client_net.listen router;
|
|
Uplink.listen uplink router
|
|
]
|
|
|
|
(* We don't use the GUI, but it's interesting to keep an eye on it.
|
|
If the other end dies, don't let it take us with it (can happen on log out). *)
|
|
let watch_gui gui =
|
|
Lwt.async (fun () ->
|
|
Lwt.try_bind
|
|
(fun () -> GUI.listen gui)
|
|
(fun `Cant_happen -> assert false)
|
|
(fun ex ->
|
|
Log.warn (fun f -> f "GUI thread failed: %s" (Printexc.to_string ex));
|
|
return ()
|
|
)
|
|
)
|
|
|
|
(* Control which of the messages that reach the reporter are logged to the console.
|
|
The rest will be displayed only if an error occurs.
|
|
Note: use the regular [Logs] configuration settings to determine which messages
|
|
reach the reporter in the first place. *)
|
|
let console_threshold _ = Logs.Info
|
|
|
|
(* Main unikernel entry point (called from auto-generated main.ml). *)
|
|
let start () =
|
|
let start_time = Clock.time () in
|
|
Logs_reporter.(create ~ring_size:20 ~console_threshold () |> run) @@ fun () ->
|
|
(* Start qrexec agent, GUI agent and QubesDB agent in parallel *)
|
|
let qrexec = RExec.connect ~domid:0 () in
|
|
let gui = GUI.connect ~domid:0 () in
|
|
let qubesDB = DB.connect ~domid:0 () in
|
|
(* Wait for clients to connect *)
|
|
qrexec >>= fun qrexec ->
|
|
let agent_listener = RExec.listen qrexec Command.handler in
|
|
gui >>= fun gui ->
|
|
watch_gui gui;
|
|
qubesDB >>= fun qubesDB ->
|
|
Log.info (fun f -> f "agents connected in %.3f s (CPU time used since boot: %.3f s)"
|
|
(Clock.time () -. start_time) (Sys.time ()));
|
|
(* Watch for shutdown requests from Qubes *)
|
|
let shutdown_rq =
|
|
OS.Lifecycle.await_shutdown_request () >>= fun (`Poweroff | `Reboot) ->
|
|
return () in
|
|
(* Set up networking *)
|
|
let net_listener = network qubesDB in
|
|
(* Report memory usage to XenStore *)
|
|
Memory_pressure.init ();
|
|
(* Run until something fails or we get a shutdown request. *)
|
|
Lwt.choose [agent_listener; net_listener; shutdown_rq] >>= fun () ->
|
|
(* Give the console daemon time to show any final log messages. *)
|
|
OS.Time.sleep 1.0
|
|
end
|