mirror of
https://github.com/eried/portapack-mayhem.git
synced 2025-07-13 01:59:49 -04:00
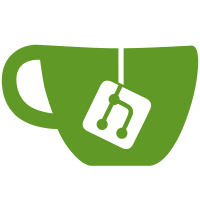
* implemented external app build * added some ui stuff for testing * added pacman game * wired key to pacman game * fixed pacman drawing issue * changed afsk rx app to be external * fixed ui::NavigationView initialization for external apps * refactoring * refactoring * moved m4 image to external app * added script for external app deployment * refactoring * implemented dynamic app listing * added color to app icon * improved app loading * added external apps to sd card content * refactoring * review findings * typo * review findings * improved memory management of bitmaps
50 lines
1.2 KiB
C++
50 lines
1.2 KiB
C++
#include "ui_pacman.hpp"
|
|
#include "irq_controls.hpp"
|
|
|
|
namespace ui::external_app::pacman {
|
|
|
|
#pragma GCC diagnostic push
|
|
// external code, so ignore warnings
|
|
#pragma GCC diagnostic ignored "-Wunused-variable"
|
|
#pragma GCC diagnostic ignored "-Wunused-parameter"
|
|
#pragma GCC diagnostic ignored "-Wunused-but-set-variable"
|
|
#pragma GCC diagnostic ignored "-Wreturn-type"
|
|
#pragma GCC diagnostic ignored "-Weffc++"
|
|
#include "playfield.hpp"
|
|
#pragma GCC diagnostic pop
|
|
|
|
Playfield _game;
|
|
|
|
PacmanView::PacmanView(NavigationView& nav)
|
|
: nav_(nav) {
|
|
add_children({&dummy});
|
|
}
|
|
|
|
void PacmanView::focus() {
|
|
dummy.focus();
|
|
}
|
|
|
|
void PacmanView::paint(Painter& painter) {
|
|
(void)painter;
|
|
|
|
if (!initialized) {
|
|
initialized = true;
|
|
_game.Init();
|
|
}
|
|
|
|
auto switches_debounced = get_switches_state().to_ulong();
|
|
|
|
but_RIGHT = (switches_debounced & 0x01) == 0x01;
|
|
but_LEFT = (switches_debounced & 0x02) == 0x02;
|
|
but_DOWN = (switches_debounced & 0x04) == 0x04;
|
|
but_UP = (switches_debounced & 0x08) == 0x08;
|
|
but_A = (switches_debounced & 0x10) == 0x10;
|
|
|
|
_game.Step();
|
|
}
|
|
|
|
void PacmanView::frame_sync() {
|
|
set_dirty();
|
|
}
|
|
|
|
} // namespace ui::external_app::pacman
|