mirror of
https://github.com/eried/portapack-mayhem.git
synced 2024-12-15 02:34:33 -05:00
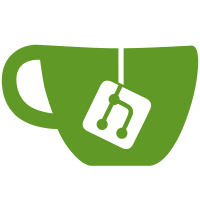
* Combined the converter from <ico>.png to bitmap.hpp and reverse in one script: pp_png2hpp.py * Minor change ficed variables from testing. * Cleanup output for parser. Add description to readme.md * Update pp_png2hpp.py Added the suggested and much cleaner argparse code from zxkmm. Added a icon-name handling, to convert one or a comma seperated subset of icons by name. * Update pp_bitmap_parser.py Updated the handler with dynamic in/outputs, in parallel to the pp_png2hpp.py script ... But I think I'll delete this one, after I decide how to handle the alpha (transparent) code.
78 lines
2.4 KiB
Python
Executable File
78 lines
2.4 KiB
Python
Executable File
#!/usr/bin/env python3
|
|
|
|
## Note: This helper was just a POC for pp_png3hpp.py to test the regex.
|
|
|
|
import argparse
|
|
import numpy as np
|
|
import re
|
|
from PIL import Image
|
|
|
|
|
|
def parse_bitmaphpp(bitmaphpp_file, icon_name):
|
|
ico_pattern = re.compile(r"static constexpr uint8_t bitmap_(.*)_data\[\] = {\n((?:\s+(?:.*)\n)+)};\nstatic constexpr Bitmap bitmap_.*\{\n\s+\{(.*)\},", re.MULTILINE)
|
|
ico_data = []
|
|
|
|
# read file to buffer, to find multiline regex
|
|
readfile = open(bitmaphpp_file,'r')
|
|
buff = readfile.read()
|
|
readfile.close()
|
|
|
|
if icon_name == 'all':
|
|
for match in ico_pattern.finditer(buff):
|
|
ico_data.append([match.group(1), match.group(2), match.group(3)])
|
|
else:
|
|
for match in ico_pattern.finditer(buff):
|
|
if match.group(1) in icon_name:
|
|
ico_data.append([match.group(1), match.group(2), match.group(3)])
|
|
|
|
return (ico_data)
|
|
|
|
def convert_hpp(icon_name,bitmap_array,iconsize_str):
|
|
iconsize = iconsize_str.split(", ")
|
|
bitmap_size = (int(iconsize[0]),int(iconsize[1]))
|
|
bitmap_data=[]
|
|
|
|
image_data = np.zeros((bitmap_size[1], bitmap_size[0]), dtype=np.uint8)
|
|
|
|
#print(bitmap_array)
|
|
for value in bitmap_array.split(",\n"):
|
|
if (value):
|
|
#print(int(value, 0))
|
|
bitmap_data.append(int(value, 0))
|
|
|
|
print(f"Count {len(bitmap_data)} Size: {bitmap_size[0]}x{bitmap_size[1]} ({bitmap_size[0]*bitmap_size[1]})")
|
|
|
|
for y in range(bitmap_size[1]):
|
|
for x in range(bitmap_size[0]):
|
|
byte_index = (y * bitmap_size[0] + x) // 8
|
|
bit_index = x % 8
|
|
# bit_index = 7 - (x % 8)
|
|
pixel_value = (bitmap_data[byte_index] >> bit_index) & 1
|
|
image_data[y, x] = pixel_value * 255
|
|
|
|
image = Image.fromarray(image_data, 'L')
|
|
imagea = image.copy()
|
|
imagea.putalpha(image)
|
|
imagea.save(icon_name+".png")
|
|
|
|
|
|
if __name__ == '__main__':
|
|
parser = argparse.ArgumentParser()
|
|
parser.add_argument("hpp", help="Path for bitmap.hpp")
|
|
parser.add_argument("--icon", help="Name of the icon from bitmap.hpp, Use 'All' for all icons in file", default = 'titlebar_image')
|
|
|
|
args = parser.parse_args()
|
|
|
|
if args.icon:
|
|
icon_name = args.icon
|
|
else:
|
|
icon_name = 'titlebar_image'
|
|
|
|
print("parse", icon_name)
|
|
icons = parse_bitmaphpp(args.hpp, icon_name)
|
|
|
|
for icon in icons:
|
|
convert_hpp(icon[0],icon[1],icon[2])
|
|
|
|
|