mirror of
https://github.com/eried/portapack-mayhem.git
synced 2024-10-01 01:26:06 -04:00
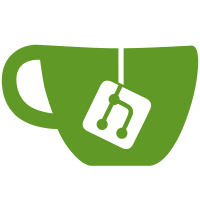
* BMP initial * Add vscode debug configuration as a template (#2109) * usb serial debug interface & usb serial async msg (#2111) * add serial_debug * not use OSS * add path print * add string print and vec * clean up * clean up * format * add an async blocking bool * add an async blocking bool - comment * protect the unexpected tx * naming * remove demo code * fix bottom-up format, and add auto extend, .. * bmp write * Minor additions * Minor * overwrite on create * Tmp * Basic view - WIP * debug * add literal str print in asyncmsg (#2113) * add literal str print in asyncmsg * remove debug things * accept suggestion per gull * fix documentary * Fix bug (#2114) * Disable Back button during Touch Calibration (#2115) * ADS1100 (#2116) * WIP * WIP * WIP * Corrected name * WIP * WIP * WIP * WIP * Added new calc * WIP * WIP * WIP * WIP * WIP * WIP * Added debug serial lines * WIP * Fixed issue * Fixed calculation issue * Added voltage to performance DFU menu * Added padding function and added voltage to perf menu * Clean up * Refactor * Fixed linting * Hides voltage if PP does not conatin IC * WIP showing battery % * made the percentage a int * Added % to header * Removed test UI * Removed comment * Added fix for precision too large * Added fix for precision too large * Linting * widget * auto zoom * remove debug * move in screen * fix math * remove test code * fix * fix compiler warning * BMP File viewer * Full screen * bg instead of noice * add comment * Handle some not supported formats. --------- Co-authored-by: E.T <tamas@eisenberger.hu> Co-authored-by: sommermorgentraum <24917424+zxkmm@users.noreply.github.com> Co-authored-by: Mark Thompson <129641948+NotherNgineer@users.noreply.github.com> Co-authored-by: jLynx <admin@jlynx.net>
70 lines
1.9 KiB
C++
70 lines
1.9 KiB
C++
/*
|
|
* Copyright (C) 2024 HTotoo
|
|
*
|
|
* This file is part of PortaPack.
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2, or (at your option)
|
|
* any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; see the file COPYING. If not, write to
|
|
* the Free Software Foundation, Inc., 51 Franklin Street,
|
|
* Boston, MA 02110-1301, USA.
|
|
*/
|
|
|
|
#ifndef __BMPFILE__H
|
|
#define __BMPFILE__H
|
|
|
|
#include <cstring>
|
|
#include <string>
|
|
|
|
#include "file.hpp"
|
|
#include "bmp.hpp"
|
|
#include "ui.hpp"
|
|
|
|
class BMPFile {
|
|
public:
|
|
~BMPFile();
|
|
bool open(const std::filesystem::path& file, bool readonly);
|
|
bool create(const std::filesystem::path& file, uint32_t x, uint32_t y);
|
|
void close();
|
|
bool is_loaded();
|
|
bool seek(uint32_t x, uint32_t y);
|
|
bool expand_y(uint32_t new_y);
|
|
bool expand_y_delta(uint32_t delta_y);
|
|
uint32_t getbpr() { return byte_per_row; };
|
|
|
|
bool read_next_px(ui::Color& px, bool seek);
|
|
bool write_next_px(ui::Color& px);
|
|
uint32_t get_real_height();
|
|
uint32_t get_width();
|
|
bool is_bottomup();
|
|
void set_bg_color(ui::Color background);
|
|
void delete_db_color();
|
|
|
|
private:
|
|
bool advance_curr_px(uint32_t num);
|
|
bool is_opened = false;
|
|
bool is_read_ony = true;
|
|
|
|
File bmpimage{};
|
|
size_t file_pos = 0;
|
|
bmp_header_t bmp_header{};
|
|
uint8_t type = 0;
|
|
uint8_t byte_per_px = 1;
|
|
uint32_t byte_per_row = 0;
|
|
|
|
uint32_t currx = 0;
|
|
uint32_t curry = 0;
|
|
ui::Color bg{};
|
|
bool use_bg = false;
|
|
};
|
|
|
|
#endif |