mirror of
https://github.com/eried/portapack-mayhem.git
synced 2024-09-20 16:15:51 +00:00
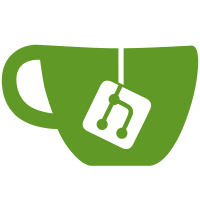
New ui_scanner, inspired on AlainD's (alain00091) PR: https://github.com/eried/portapack-mayhem/pull/80 It includes the following: 1) A big frequency numbers display. 2) A Manual scan section (you can input a frequency range (START / END), choose a STEP value from an available of standard frequency intervals, and press SCAN button. 3) An AM / WFM / NFM scan mode selector, changing "on the fly". 4) A PAUSE / RESUME button, which will make the scanner to stop upon you listening something of interest 5) AUDIO APP button, a quick shortcut into the analog audio visualizing / recording app, with the mode, frequency, amp, LNA, VGA settings already in tune with the scanner. 6) Two enums are added to freqman.hpp, reserved for compatibility with AlainD's proposed freqman's app and / or further enhancement. More on this topic: ORIGINAL scanner just used one frequency step, when creating scanning frequency ranges, which was unacceptable. AlainD enhanced freqman in order to pass different steppings along with ranges. This seems an excellent idea, and I preserved that aspect on my current implementation of thisscanner, while adding those enums into the freqman just to keep the door open for AlainD's freqman in the future. 7) I did eliminate the extra blank spaces added by function to_string_short_freq() which created unnecessary spacing in every app where there is need for a SHORT string, from a frequency number. (SHORT!, no extra spaces!!) 8) I also maintained AlainD idea of capping the number of frequencies which are dynamically created for each range and stored inside a memory based db. While AlainD capped the number into 400 frequencies, I was able to up that value a bit more, into 500. Cheers!
84 lines
2.3 KiB
C++
84 lines
2.3 KiB
C++
/*
|
|
* Copyright (C) 2014 Jared Boone, ShareBrained Technology, Inc.
|
|
* Copyright (C) 2016 Furrtek
|
|
*
|
|
* This file is part of PortaPack.
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2, or (at your option)
|
|
* any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; see the file COPYING. If not, write to
|
|
* the Free Software Foundation, Inc., 51 Franklin Street,
|
|
* Boston, MA 02110-1301, USA.
|
|
*/
|
|
|
|
#include <cstring>
|
|
#include <string>
|
|
#include "file.hpp"
|
|
#include "ui_receiver.hpp"
|
|
#include "string_format.hpp"
|
|
|
|
#ifndef __FREQMAN_H__
|
|
#define __FREQMAN_H__
|
|
|
|
#define FREQMAN_DESC_MAX_LEN 30
|
|
#define FREQMAN_MAX_PER_FILE 99
|
|
#define FREQMAN_MAX_PER_FILE_STR "99"
|
|
|
|
using namespace ui;
|
|
using namespace std;
|
|
|
|
enum freqman_error {
|
|
NO_ERROR = 0,
|
|
ERROR_ACCESS,
|
|
ERROR_NOFILES,
|
|
ERROR_DUPLICATE
|
|
};
|
|
|
|
enum freqman_entry_type {
|
|
SINGLE = 0,
|
|
RANGE
|
|
};
|
|
|
|
//Entry step placed for AlainD freqman version (or any other enhanced version)
|
|
enum freqman_entry_step {
|
|
STEP_DEF = 0, // default
|
|
AM_US, // 10 Khz AM/CB
|
|
AM_EUR, // 9 Khz LW/MW
|
|
NFM_1, // 12,5 Khz (Analogic PMR 446)
|
|
NFM_2, // 6,25 Khz (Digital PMR 446)
|
|
FM_1, // 100 Khz
|
|
FM_2, // 50 Khz
|
|
N_1, // 25 Khz
|
|
N_2, // 250 Khz
|
|
AIRBAND, // AIRBAND 8,33 Khz
|
|
ERROR_STEP
|
|
};
|
|
|
|
// freqman_entry_step step added, as above, to provide compatibility / future enhancement.
|
|
struct freqman_entry {
|
|
rf::Frequency frequency_a { 0 };
|
|
rf::Frequency frequency_b { 0 };
|
|
std::string description { };
|
|
freqman_entry_type type { };
|
|
freqman_entry_step step { };
|
|
};
|
|
|
|
using freqman_db = std::vector<freqman_entry>;
|
|
|
|
std::vector<std::string> get_freqman_files();
|
|
bool load_freqman_file(std::string& file_stem, freqman_db& db);
|
|
bool save_freqman_file(std::string& file_stem, freqman_db& db);
|
|
bool create_freqman_file(std::string& file_stem, File& freqman_file);
|
|
std::string freqman_item_string(freqman_entry &item, size_t max_length);
|
|
|
|
#endif/*__FREQMAN_H__*/
|