mirror of
https://github.com/keepassxreboot/keepassxc.git
synced 2024-10-01 01:26:01 -04:00
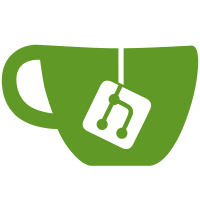
Many lines were not conformant with the project's formatting rules. This patch should fix all formatting and whitespace issues in the code base. A clang-format directive was put around the connect() calls containing SIGNALs and SLOTs whose signatures would be denormalized because of the formatting rules.
64 lines
2.0 KiB
C++
64 lines
2.0 KiB
C++
/*
|
|
* Copyright (C) 2017 KeePassXC Team <team@keepassxc.org>
|
|
*
|
|
* This program is free software: you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation, either version 2 or (at your option)
|
|
* version 3 of the License.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
|
*/
|
|
|
|
#ifndef KEEPASSXC_ASYNCTASK_HPP
|
|
#define KEEPASSXC_ASYNCTASK_HPP
|
|
|
|
#include <QFuture>
|
|
#include <QFutureWatcher>
|
|
#include <QtConcurrent>
|
|
|
|
/**
|
|
* Asynchronously run computations outside the GUI thread.
|
|
*/
|
|
namespace AsyncTask
|
|
{
|
|
|
|
/**
|
|
* Wait for the given future without blocking the event loop.
|
|
*
|
|
* @param future future to wait for
|
|
* @return async task result
|
|
*/
|
|
template <typename FunctionObject>
|
|
typename std::result_of<FunctionObject()>::type
|
|
waitForFuture(QFuture<typename std::result_of<FunctionObject()>::type> future)
|
|
{
|
|
QEventLoop loop;
|
|
QFutureWatcher<typename std::result_of<FunctionObject()>::type> watcher;
|
|
QObject::connect(&watcher, SIGNAL(finished()), &loop, SLOT(quit()));
|
|
watcher.setFuture(future);
|
|
loop.exec();
|
|
return future.result();
|
|
}
|
|
|
|
/**
|
|
* Run a given task and wait for it to finish without blocking the event loop.
|
|
*
|
|
* @param task std::function object to run
|
|
* @return async task result
|
|
*/
|
|
template <typename FunctionObject>
|
|
typename std::result_of<FunctionObject()>::type runAndWaitForFuture(FunctionObject task)
|
|
{
|
|
return waitForFuture<FunctionObject>(QtConcurrent::run(task));
|
|
}
|
|
|
|
}; // namespace AsyncTask
|
|
|
|
#endif // KEEPASSXC_ASYNCTASK_HPP
|