mirror of
https://github.com/keepassxreboot/keepassxc.git
synced 2024-09-20 16:15:44 +00:00
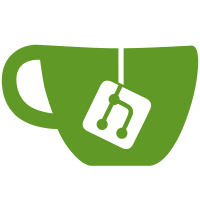
This change adds a GNU Readline-based interactive mode to keepassxc-cli. If GNU Readline is not available, commands are just read from stdin with no editing or auto-complete support. DatabaseCommand is modified to add the path to the current database to the arguments passed to executeWithDatabase. In this way, instances of DatabaseCommand do not have to prompt to re-open the database after each invocation, and existing command implementations do not have to be changed to support interactive mode. This change also introduces a new way of handling commands between interactive and batch modes. * Fixes #3224. * Ran make format
97 lines
2.7 KiB
C++
97 lines
2.7 KiB
C++
/*
|
|
* Copyright (C) 2019 KeePassXC Team <team@keepassxc.org>
|
|
*
|
|
* This program is free software: you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation, either version 2 or (at your option)
|
|
* version 3 of the License.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
|
*/
|
|
|
|
#ifndef KEEPASSXC_TESTCLI_H
|
|
#define KEEPASSXC_TESTCLI_H
|
|
|
|
#include "core/Database.h"
|
|
#include "util/TemporaryFile.h"
|
|
|
|
#include <QByteArray>
|
|
#include <QFile>
|
|
#include <QScopedPointer>
|
|
#include <QSharedPointer>
|
|
#include <QTemporaryFile>
|
|
#include <QTest>
|
|
|
|
#include <stdio.h>
|
|
|
|
class TestCli : public QObject
|
|
{
|
|
Q_OBJECT
|
|
|
|
private:
|
|
QSharedPointer<Database> readTestDatabase() const;
|
|
|
|
private slots:
|
|
void initTestCase();
|
|
void init();
|
|
void cleanup();
|
|
void cleanupTestCase();
|
|
|
|
void testBatchCommands();
|
|
void testAdd();
|
|
void testAddGroup();
|
|
void testAnalyze();
|
|
void testClip();
|
|
void testCommandParsing_data();
|
|
void testCommandParsing();
|
|
void testCreate();
|
|
void testDiceware();
|
|
void testEdit();
|
|
void testEstimate_data();
|
|
void testEstimate();
|
|
void testExport();
|
|
void testGenerate_data();
|
|
void testGenerate();
|
|
void testKeyFileOption();
|
|
void testNoPasswordOption();
|
|
void testHelp();
|
|
void testInteractiveCommands();
|
|
void testList();
|
|
void testLocate();
|
|
void testMerge();
|
|
void testMove();
|
|
void testOpen();
|
|
void testRemove();
|
|
void testRemoveGroup();
|
|
void testRemoveQuiet();
|
|
void testShow();
|
|
void testInvalidDbFiles();
|
|
void testYubiKeyOption();
|
|
|
|
private:
|
|
QByteArray m_dbData;
|
|
QByteArray m_dbData2;
|
|
QByteArray m_yubiKeyProtectedDbData;
|
|
QByteArray m_keyFileProtectedDbData;
|
|
QByteArray m_keyFileProtectedNoPasswordDbData;
|
|
QScopedPointer<TemporaryFile> m_dbFile;
|
|
QScopedPointer<TemporaryFile> m_dbFile2;
|
|
QScopedPointer<TemporaryFile> m_keyFileProtectedDbFile;
|
|
QScopedPointer<TemporaryFile> m_keyFileProtectedNoPasswordDbFile;
|
|
QScopedPointer<TemporaryFile> m_yubiKeyProtectedDbFile;
|
|
QScopedPointer<TemporaryFile> m_stdoutFile;
|
|
QScopedPointer<TemporaryFile> m_stderrFile;
|
|
QScopedPointer<TemporaryFile> m_stdinFile;
|
|
FILE* m_stdoutHandle = stdout;
|
|
FILE* m_stderrHandle = stderr;
|
|
FILE* m_stdinHandle = stdin;
|
|
};
|
|
|
|
#endif // KEEPASSXC_TESTCLI_H
|